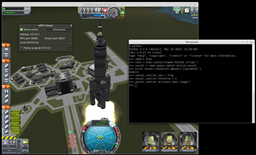
Expose career info like Funds, Reputation and Science as well as the current GameMode
lucaelin opened this issue ยท 3 comments
It would be useful to expose Funding.Instance.Funds
, Reputation.Instance.reputation
and ResearchAndDevelopment.Instance.Science
if the current game is either in science or career mode.
It would be great to add the actual HighLogic.CurrentGame.Mode
to the API as well.
I have no clue about C#, but i've designed a prove of concept like this:
SpaceCenter.cs
/// <summary>
/// The current mode the game is in.
/// </summary>
[KRPCProperty]
public static GameMode GameMode
{
get
{
if (HighLogic.CurrentGame.Mode == Game.Modes.CAREER)
return GameMode.Career;
if (HighLogic.CurrentGame.Mode == Game.Modes.SCIENCE_SANDBOX)
return GameMode.Science;
if (HighLogic.CurrentGame.Mode == Game.Modes.SANDBOX)
return GameMode.Sandbox;
return GameMode.Scenario;
}
}
GameMode.cs
using System;
using KRPC.Service.Attributes;
namespace KRPC.SpaceCenter
{
/// <summary>
/// The game mode.
/// Returned by <see cref="GameMode"/>
/// </summary>
[Serializable]
[KRPCEnum(Service = "SpaceCenter")]
public enum GameMode
{
/// <summary>
/// The game is in sandbox mode.
/// </summary>
Sandbox,
/// <summary>
/// The game is in science mode.
/// </summary>
Science,
/// <summary>
/// The game is in career mode.
/// </summary>
Career,
/// <summary>
/// The game is in scenario mode.
/// </summary>
Scenario
}
}
Might as well add the rest of my code:
SpaceCenter.cs
/// <summary>
/// The current amount of science.
/// </summary>
[KRPCProperty]
public static float Science
{
get {
if (ReferenceEquals(ResearchAndDevelopment.Instance, null))
throw new InvalidOperationException("Science not available");
return ResearchAndDevelopment.Instance.Science;
}
}
/// <summary>
/// The current amount of funds.
/// </summary>
[KRPCProperty]
public static double Funds {
get {
if (ReferenceEquals(Funding.Instance, null))
throw new InvalidOperationException("Funding not available");
return Funding.Instance.Funds;
}
}
/// <summary>
/// The current amount of reputation.
/// </summary>
[KRPCProperty]
public static float Reputation
{
get {
if (ReferenceEquals(global::Reputation.Instance, null))
throw new InvalidOperationException("Reputation not available");
return global::Reputation.Instance.reputation;
}
}