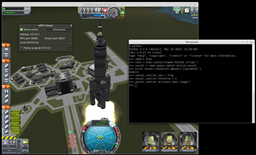
Python code linting and static type checking
djungelorm opened this issue ยท 1 comments
Consider updating the build system to use:
- black for code formatting, replacing pycodestyle. It's more modern, and can auto update source files.
- pylint for code analysis (we already use this)
- mypy for static type checking (and add python code type annotations)
For mypy, I have tested the code locally and it passes using the following script. This runs mypy in strict mode on client with and without generated service stubs.
#!/bin/bash
set -ev
bazel build //client/python:python
env/bin/pip install --upgrade bazel-bin/client/python/krpc-0.5.1.zip
env/bin/python -m mypy --config-file client/python/mypy.ini test.py client/python/krpc/
env/bin/pip install --upgrade bazel-bin/client/python/krpc-base-0.5.1.zip
env/bin/python -m mypy --config-file client/python/mypy-base.ini test-base.py client/python/krpc/
client/python/mypy.ini
[mypy]
strict = True
[mypy-krpc.services.*]
disable_error_code = misc, assignment, return-value
client/python/mypy-base.ini
[mypy]
strict = True
[mypy-krpc.client]
warn_unused_ignores = False
[mypy-krpc.services.*]
disable_error_code = import
test.py
import krpc
from krpc.client import Client
from krpc.services.spacecenter import Vessel
def run(conn: Client) -> None:
sc = conn.space_center
v: Vessel = sc.active_vessel
conn = krpc.connect(use_pregenerated_stubs=False)
run(conn)
conn = krpc.connect(use_pregenerated_stubs=True)
run(conn)
test-base.py
import krpc
from krpc.client import Client
def run(conn: Client) -> None:
sc = conn.space_center
v = sc.active_vessel
conn = krpc.connect(use_pregenerated_stubs=False)
run(conn)
conn = krpc.connect(use_pregenerated_stubs=True)
run(conn)