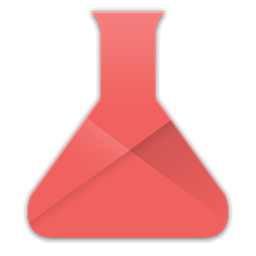
[1.13] Replace items.csv with ItemCodexLib (items.json w/ 100% coverage).
dscalzi opened this issue ยท 15 comments
Related: #1906 (can be closed).
Hello everyone, I've spent a non-trivial amount of time over the past year writing conversion scripts to convert the items.csv file to JSON while preserving all the data. When 1.13 dropped I converted everything to be 1.13 compliant. The culmination of this effort is my library ItemCodexLib.
It serves as the replacement for items.csv, making everything easier than its ever been. With the library, you can easily interact with the json file through a straightforward API. The library is small and lightweight, just shade + relocate it into the final jar file.
The JSON file has 100% of 1.13. All new items have been added and old aliases have been cleaned up. The file is extensive, so if we did miss anything simply point it out and it will be corrected.
Potions are 100% supported.
Repo:
https://github.com/dscalzi/ItemCodexLib
Example API Usage:
public void itemCodexExample() {
// Load the items file and setup the mappings.
ItemCodex codex = new ItemCodex(this.plugin);
// Get stone by its material name.
codex.getItem("STONE").ifPresent((entry) -> {
ItemStack stone = entry.getItemStack();
});
// Get stone by an alias.
codex.getItem("rock").ifPresent((entry) -> {
ItemStack stone = entry.getItemStack();
});
// Get stone by its legacy id.
codex.getItem("1").ifPresent((entry) -> {
ItemStack stone = entry.getItemStack();
});
// Get stone by its legacy id and data value.
// Let's use a different Optional construct.
Optional<ItemEntry> entryOptional = codex.getItem("1:0");
if(entryOptional.isPresent()) {
ItemStack stone = entryOptional.get().getItemStack();
}
}
You can install with maven.
<repository>
<id>jcenter</id>
<name>jcenter-bintray</name>
<url>http://jcenter.bintray.com</url>
</repository>
<dependency>
<groupId>com.dscalzi</groupId>
<artifactId>ItemCodexLib</artifactId>
<version>VERSION</version>
</dependency>
Details on the item format:
Charcoal (no NBT data).
{
"spigot": {
"material": "CHARCOAL"
},
"legacy": {
"id": 263,
"data": 1
},
"aliases": [
"charcoal",
"ccoal"
]
}
Lingering Potion of Strength II (NBT data).
{
"spigot": {
"material": "LINGERING_POTION",
"potionData": {
"type": "STRENGTH",
"extended": false,
"upgraded": true
}
},
"legacy": {
"id": 441,
"data": 0
},
"aliases": [
"lingerpotstrengthii",
"strengthlingerpotii",
"lingerpotstrii",
"strlingerpotii"
]
}
I am using this library in my plugin VirtualShopX, which works wonderfully on 1.13
https://github.com/dscalzi/VirtualShopX
If you'd like, I could see about submitting a PR to add support for this. I am making the issue first because I'd rather not spend all that time for no reason.
Supports 1.13.1 items.
How do i get this to work with 1.13.2 Spigot Server? i use EssentialsX-2.16.0.32.jar
I am a noob at Java and other scripting but i just cant get the items.json to work
@bjornstevens I'm not sure what you're trying to do, but this issue was for a rejected proposal for a different items database.
If you're running into an issue using EssentialsX, please open a new issue.
@bjornstevens I'm not sure what you're trying to do, but this issue was for a rejected proposal for a different items database.
If you're running into an issue using EssentialsX, please open a new issue.
Let me try to tell my situation:
I have a shop with more than 400 signs, the signs have item id's on them (like 138 for beacon and 189 for birch fence). I just updated the server last sunday to 1.13.2 (and all the plugins) but when i tried to use the buy and sell sign it says: error unknown item name.
I thought this ItemCodexLib would be able to fix my issue. But i dont know how to use it...
Server info:
OS: Linux Mint x64
Spigot: Spigot 1.13.2
EssentialsX: EssentialsX-2.16.0.32.jar
Extra:
All installed plugins (in red are installed and in use)
Edit by @md678685: removed personal information
@bjornstevens ItemCodexLib has nothing to do with EssentialsX. This thread is a closed feature request, not a place to ask for support.
There are no item IDs in 1.13 or above. You need to use item names on signs now, not IDs.
As I stated before, if you're running into an issue using EssentialsX, please open a new issue.
@dscalzi The "shop plugin" in question is in fact EssentialsX signs. IDs are not supported in Minecraft 1.13 and we have no intention of upholding a convention that has been discouraged for years and is becoming increasingly antiquated and irrelevant.
@bjornstevens
If you're the author of the shop plugin, implement it with ItemCodeLib. If you're not the author, tell them to update to 1.13 and send them this link. https://github.com/dscalzi/ItemCodexLib
Can i then use the short names?
yes, i can haha
i will now start to change the signs to the shorter names, hopefully those never change.
@dscalzi The "shop plugin" in question is in fact EssentialsX signs. IDs are not supported in Minecraft 1.13 and we have no intention of upholding a convention that has been discouraged for years and is becoming increasingly antiquated and irrelevant.
Ah okey, thanks for the info!
Luckely i have a plugin installed to edit signs so i just have to look up on the 1.12.2 item ID list.
Wish they still had implemented Item ID's in the 1.13 (and above) versions. At least not i don't have to search further.
Thanks for the fast replies @md678685 and @dscalzi
@bjornstevens You can hold the item and run /itemdb
, or just run /itemdb <item name>
to list all of EssentialsX's supported names for items.
@bjornstevens You can hold the item and run
/itemdb
, or just run/itemdb <item name>
to list all of EssentialsX's supported names for items.
Ah thanks!
Can i then use the short names?
+1. So useful and efficient, there is no real need to work on a different one, using this will save so much time for you guys.
Thanks for the offer, however at present we're not intending to gut the reliable tested ItemDb code inherited from Essentials and the providers implemented early on in the EssentialsX project. Instead, we're intending to handle this entirely in-house using a toolkit we've already invested a large amount of time in, maintaining the items.csv
as a fallback until we can no longer reasonably support it.
The library looks quite handy, but we're not planning to incorporate additional third-party libraries into the main project.
ItemCodexLib now has full support for 1.8.x, 1.9.x, 1.10.x, 1.11.x, 1.12.x, and 1.13.x.
Potions are also fully supported for 1.8-1.13.
Almost a month later.. any update? @md678685