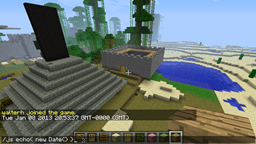
How can I type cast java object
maple3142 opened this issue ยท 2 comments
as title
events.entityDamage(function(e){
var en=e.getEntity();
console.log(en);
var LivingEntity=org.bukkit.entity.LivingEntity;
if(en instanceof LivingEntity){
en=(org.bukkit.entity.LivingEntity)en;//error
if(e.getDamage()>en.getHealth()){
e.setDamage(0);
}
}
});
I haven't tried this online but try this version of your code:
events.entityDamage(function(e){
var en=e.getEntity();
console.log(en);
var LivingEntity=Java.type("org.bukkit.entity.LivingEntity");
if(en instanceof LivingEntity){
en=Java.to(en,LivingEntity);
if(e.getDamage()>en.getHealth()){
e.setDamage(0);
}
}
});
I'm curious if you could save a line and do it like this:
events.entityDamage(function(e){
var LivingEntity=Java.type("org.bukkit.entity.LivingEntity");
var en=Java.to( e.getEntity() , LivingEntity ); // could throw exception?
console.log(en); // how does this render?
if(en instanceof LivingEntity){ // unnecessary now that it's already cast?
if(e.getDamage()>en.getHealth()){
e.setDamage(0);
}
}
});
If the check for instanceof
isn't necessary then you can eliminate three more lines:
var en=Java.to( e.getEntity() , Java.type("org.bukkit.entity.LivingEntity") );
Related tips:
https://docs.oracle.com/javase/8/docs/technotes/guides/scripting/nashorn/api.html
https://docs.oracle.com/javase/8/docs/technotes/guides/scripting/prog_guide/javascript.html
Please let me know how this works for ya!
For questions that aren't actually issues with the software, I recommend the Google Group, where more people can see your notes.
https://groups.google.com/forum/?fromgroups#!forum/scriptcraft---scripting-minecraft
HTH
You don't need to typecast - Javascript is dynamic.
events.entityDamage(function(evt){
// does evt.entity object have a 'health' property ? if so it's a living entity
if ('health' in evt.entity) {
if ( evt.damage > evt.entity.health ) {
evt.damage = 0;
}
}
});
You don't need to use .getHealth()
or .getDamage()
- they're Javabean properties so they can be accessed in Javascript using simply .health
and .damage