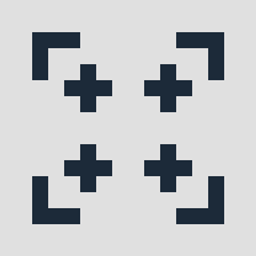
Chunk data label:question
dragonflame86 opened this issue ยท 5 comments
Ok so I'm trying to use cardinal components to store data onto a chunk,
Which I can then grab at any time. In this instance I am trying to store 2 double values to a chunk lets call them X and Y
public interface DoubleComponent extends ComponentV3 {
double getX();
void setX(double value);
double getY();
void setY(double value);
}
public class ChunkDoubleComponent implements VisComponent {
public double x = (Math.random() * 50);
public double y = (Math.random() * 20);
@Override
public void readFromNbt(CompoundTag compoundTag) {
this.x= compoundTag.getDouble("xdata");
this.y = compoundTag.getDouble("ydata");
}
@Override
public void writeToNbt(CompoundTag compoundTag) {
compoundTag.putDouble("xdata", this.x);
compoundTag.putDouble("ydata", this.y);
}
@Override
public double getX() { return this.x; }
@Override
public double getY() { return this.y; }
@Override
public void setX(double x) { this.x = x; }
@Override
public void setY(double y) { this.y = y; }
}
They are stored just fine I can come back into a chunk and they remain
but the moment I restart the world, their data disappears, is this intentional or am I doing something horribly wrong and am just unaware?
ok, so I am going to assume you mean the physical code for registration
public static final ComponentKey<ChunkDoubleComponent> XY = ComponentRegistryV3.INSTANCE.getOrCreate(new Identifier(MyMod.MOD_ID,"vis"), ChunkDoubleComponent.class);
@Override
public void registerChunkComponentFactories(ChunkComponentFactoryRegistry registry) {
registry.register(XY, chunk -> new ChunkDoubleComponent());
}
as for game log after rejoining the world there is nothing really there out of the ordinary
[22:14:28] [main/INFO] (Minecraft) Loaded 8 recipes
[22:14:28] [main/INFO] (Minecraft) Loaded 927 advancements
[22:14:28] [main/INFO] (BiomeModificationImpl) Applied 0 biome modifications to 0 of 79 new biomes in 4.328 ms
[22:14:29] [main/INFO] (Minecraft) Environment: authHost='https://authserver.mojang.com', accountsHost='https://api.mojang.com', sessionHost='https://sessionserver.mojang.com', servicesHost='https://api.minecraftservices.com', name='PROD'
[22:14:29] [main/INFO] (Portal) IP Config Applied
[22:14:29] [Server thread/INFO] (Minecraft) Starting integrated minecraft server version 1.16.5
[22:14:29] [Server thread/INFO] (Minecraft) Generating keypair
[22:14:30] [Server thread/INFO] (Portal) Successfully read IP's dimension id record
[22:14:30] [Server thread/INFO] (Portal) Start Completing Dimension Id Record
[22:14:30] [Server thread/INFO] (Portal) Before:
minecraft:overworld -> 0
minecraft:the_nether -> -1
minecraft:the_end -> 1
[22:14:30] [Server thread/INFO] (Portal) Server Loaded Dimensions:
minecraft:overworld
minecraft:the_end
minecraft:the_nether
[22:14:30] [Server thread/INFO] (Portal) After:
minecraft:overworld -> 0
minecraft:the_nether -> -1
minecraft:the_end -> 1
[22:14:30] [Server thread/INFO] (Portal) Dimension Id Info Saved to File
[22:14:30] [Server thread/INFO] (Minecraft) Preparing start region for dimension minecraft:overworld
[22:14:31] [main/INFO] (Minecraft) Preparing spawn area: 0%
[22:14:31] [main/INFO] (Minecraft) Preparing spawn area: 0%
[22:14:31] [main/INFO] (Minecraft) Preparing spawn area: 0%
[22:14:31] [Server thread/INFO] (Portal) Loading custom portal gen
[22:14:31] [main/INFO] (Minecraft) Time elapsed: 1454 ms
[22:14:32] [Server thread/INFO] (Minecraft) Changing view distance to 11, from 10
[22:14:33] [Server thread/INFO] (Minecraft) Player957[local:E:87fbac78] logged in with entity id 248 at (1.8645500774731123, 104.06251836166818, -85.13628354930958)
[22:14:33] [Server thread/INFO] (Minecraft) Player957 joined the game
[22:14:33] [Netty Local Client IO #0/INFO] (Portal) Received Dimension Type Sync
[22:14:33] [Netty Local Client IO #0/INFO] (Portal)
minecraft:overworld -> minecraft:overworld
minecraft:the_nether -> minecraft:the_nether
minecraft:the_end -> minecraft:the_end
[22:14:33] [Netty Local Client IO #0/INFO] (Portal) Received Dimension Int Id Sync
[22:14:33] [Netty Local Client IO #0/INFO] (Portal)
minecraft:overworld -> 0
minecraft:the_nether -> -1
minecraft:the_end -> 1
[22:14:34] [main/INFO] (Portal) WorldRenderer reloaded minecraft:overworld
[22:14:34] [main/WARN] (Minecraft) Unknown recipe category: my_mod_craft:basic_infuser_recipe/my_mod_craft:portable_hole
[22:14:34] [Server thread/INFO] (Minecraft) Saving and pausing game...
[22:14:34] [Server thread/INFO] (Minecraft) Saving chunks for level 'ServerWorld minecraft:overworld test i guess'/minecraft:overworld
[22:14:34] [Server thread/INFO] (Minecraft) Saving chunks for level 'ServerWorld minecraft:the_nether test i guess'/minecraft:the_nether
[22:14:34] [Server thread/INFO] (Minecraft) Saving chunks for level 'ServerWorld minecraft:the_end test i guess'/minecraft:the_end
[22:14:35] [main/INFO] (Minecraft) Loaded 0 advancements
[22:14:41] [Server thread/INFO] (Minecraft) Saving and pausing game...
[22:14:41] [Server thread/INFO] (Minecraft) Saving chunks for level 'ServerWorld minecraft:overworld test i guess'/minecraft:overworld
[22:14:42] [Server thread/INFO] (Minecraft) Saving chunks for level 'ServerWorld minecraft:the_nether test i guess'/minecraft:the_nether
[22:14:42] [Server thread/INFO] (Minecraft) Saving chunks for level 'ServerWorld minecraft:the_end test i guess'/minecraft:the_end
[22:18:04] [Server thread/INFO] (Minecraft) Player957 lost connection: Disconnected
[22:18:04] [Server thread/INFO] (Minecraft) Player957 left the game
[22:18:04] [Server thread/INFO] (Minecraft) Stopping singleplayer server as player logged out
[22:18:04] [Server thread/INFO] (Minecraft) Stopping server
[22:18:04] [Server thread/INFO] (Minecraft) Saving players
[22:18:04] [Server thread/INFO] (Minecraft) Saving worlds
[22:18:04] [Server thread/INFO] (Minecraft) Saving chunks for level 'ServerWorld minecraft:overworld test i guess'/minecraft:overworld
[22:18:06] [Server thread/INFO] (Minecraft) ThreadedAnvilChunkStorage (test i guess): All chunks are saved
[22:18:06] [Server thread/INFO] (Minecraft) Saving chunks for level 'ServerWorld minecraft:the_nether test i guess'/minecraft:the_nether
[22:18:06] [Server thread/INFO] (Minecraft) ThreadedAnvilChunkStorage (DIM-1): All chunks are saved
[22:18:06] [Server thread/INFO] (Minecraft) Saving chunks for level 'ServerWorld minecraft:the_end test i guess'/minecraft:the_end
[22:18:06] [Server thread/INFO] (Minecraft) ThreadedAnvilChunkStorage (DIM1): All chunks are saved
[22:18:06] [Server thread/INFO] (Minecraft) ThreadedAnvilChunkStorage (test i guess): All chunks are saved
[22:18:06] [Server thread/INFO] (Minecraft) ThreadedAnvilChunkStorage (DIM-1): All chunks are saved
[22:18:06] [Server thread/INFO] (Minecraft) ThreadedAnvilChunkStorage (DIM1): All chunks are saved
[22:18:16] [main/INFO] (Minecraft) Stopping!
Hm, I am not seeing anything immediately wrong here, are you modifying the values clientside maybe ?
Last I checked I am literally just reading them once I open the world or load a new chunk
double x = ChunkGeneration.XY.maybeGet(chunk).map(ChunkGeneration.ChunkDoubleComponent::getX).orElse(0.1);
double y = ChunkGeneration.XY.maybeGet(chunk).map(ChunkGeneration.ChunkDoubleComponent::getY).orElse(0.1);
Is there a chance that reading these on the client side may cause an issue?
i am unsure if i am calling these checks on the clientside(i'll have to check)
but in case i am, chances are im reading these values for good reason
is there a way to read them on the clientside without this issue?
If you are reading the values too early (eg. in a constructor), the deserialization may not have happened yet, even serverside. If you are reading values clientside, you can add the AutoSyncedComponent interface to your component.