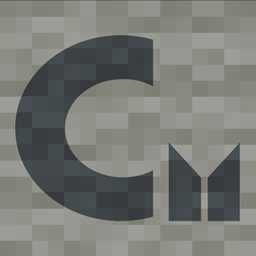
[Suggestion] property -> state
fghsgh opened this issue ยท 4 comments
I am trying to write a Scarpet app that can rotate/mirror blocks similarly to how structure blocks do it, but I seem to be unable to find a way to read or process the block states of a block. Ideally, I suggest making the block object be accessible as if it were a map, with the :
operator, but also with all functions that work on maps, like keys()
and get()
:
b = block(100,100,100); // get block at position 100 100 100 (assume this block is 'redstone_wire[east=none,north=none,power=0,south=up,west=side]')
print(b); // says 'redstone_wire'
print(b:'east'); // says 'none'
print(b:'power') // says '0' (for clarity, this is a string with the value '0', not a number, although that is also possible)
Furthermore, it needs to be possible to modify these block states (potentially requiring for the block object to be a "dummy" block as is obtained by passing a string to block()
):
b:'east' = 'up';
Then, the block with modified block states can be placed somewhere else using set()
.
Alternatively, a function getstates(block)
could be implemented which returns the states of the given block as a map, so that it can be read and modified by the script. Alternatively, any other way of accessing the entire block states is also possible (preferably one that can be iterated over, as is possible with maps).
EDIT:
Additionally, it would be nice if there was a method for rotating/mirroring block states easily, since it seems Minecraft can already do this (structure blocks), so this method could be implemented relatively easily, but also because it would probably be less efficient in Scarpet anyway.
It appears that, although "block states", or any variant thereof, does not appear in the documentation in any helpful places, there are the methods block_properties()
and property()
which together can be used to access all block states of a block. Why they are called "block properties" rather than block states seems like a mystery to me, as they are called block states everywhere else, including on Minecraft Wiki. Thus, as it appears, my original suggestion was already fulfilled before I made it. Hence I retract the above suggestion and instead I request that "block states" be at least mentioned in the documentation under the aforementioned functions, for the sake of accessibility. This does not require barely any effort, so I feel like it is a reasonable request.
well, the value of the state is called a property, so technically block_properties
should be called block_states
, but property
should stay. This excerpt is taken from the code using Mojang mappings for example
private <T extends Comparable<T>> void validateProperty(Property<T> lvt1) {
String s = lvt1.getName();
if (!StateDefinition.NAME_PATTERN.matcher(s).matches()) {
throw new IllegalArgumentException(this.owner + " has invalidly named property: " + s);
} else {
Collection<T> collection = lvt1.getPossibleValues();
if (collection.size() <= 1) {
throw new IllegalArgumentException(this.owner + " attempted use property " + s + " with <= 1 possible values");
} else {
for(T t : collection) {
String s1 = lvt1.getName(t);
if (!StateDefinition.NAME_PATTERN.matcher(s1).matches()) {
throw new IllegalArgumentException(this.owner + " has property: " + s + " with invalidly named value: " + s1);
}
}
if (this.properties.containsKey(s)) {
throw new IllegalArgumentException(this.owner + " has duplicate property: " + s);
}
}
}
}
Used that ticket as a pretext for this change. The main reason is that I wanted block_properties to return a map, not a list, but maps were not part of the scarpet when block_properties were introduced. To properly deprecate it, I needed to use a different name an block_state was relevant.