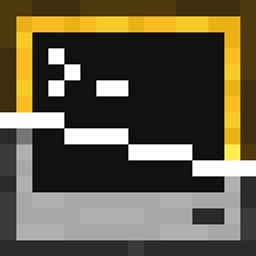
Felling Turtle Gets Stuck
octagonalsquare opened this issue ยท 3 comments
Useful information to include:
- Minecraft version: 1.18.1
- CC: Restitched version: 1.100.1 (experienced on 1.100.0 first then updated to see if it was fixed)
- Logs: none
The felling turtle gets stuck randomly. I am testing a new program so I am not leaving the area and unloading the chunk (plus its in spawn chunks), it has fuel and knows how to refuel, and there are no unbreakable blocks in its way. So I have a function that looks like this:
`function fw(l)
l=l or 1
for i=1, l do
local tries = 0
while turtle.forward() ~= true do
turtle.dig()
turtle.attack()
sleep(0.2)
tries = tries+1
if tries > 500 then
printError("Can't move forward")
return false
end
end
end
return true
end`
It allows you to call fw(distance) and it will move the turtle forward "distance" many blocks, breaking blocks and killing things along the way. If it can't move forward, it tries to break a block, then tries to kill anything in the way, waits .2 seconds, and then tries again to move forward. What is happening is the turtle will be moving forward, have nothing in the way, and get stuck because "turtle.forward()" is returning false, meaning it can't move forward.
My attempt to remedy it was to have it try to go around the "blockage" in front by changing the function to this:
`function fw(l)
l=l or 1
for i=1, l do
local tries = 0
while turtle.forward() ~= true do
turtle.dig()
turtle.attack()
sleep(0.2)
tries = tries+1
if tries > 0 then
printError("Can't move forward")
turtle.turnRight()
fw()
turtle.turnLeft()
fw(2)
turtle.turnLeft()
fw()
turtle.TurnRight()
i = i+2
end
end
end
return true
end`
this should make it go around that block and set the loop properly. But instead it spins as if it is blocked on all four sides.
I have not seen this happen with other turtles. I use a mining turtle for a quarry. The quarry file also uses this function for movement and it doesn't get stuck except when the chunk unloads. To replicate:
- make a felling turtle
- type
pastebin get s7UrhTnG setup
- run setup
- then run trees
The file makes the turtle go around a predetermined path to cut down trees so it moves weird, but it will go around, wait a few seconds and go again. I've noticed it happens most when it runs right after I log on or if it has run several times. The one thing I've found is that if I place a block where it thinks its being stuck, then reset the turtle to its starting point and run it again, it works fine
Tried running it on a mining turtle and it also gets stuck, so its not just felling turtles
I noticed that if I terminated the program and restarted it where the turtle was, it would no longer be stuck, but running a different program it would still be stuck. So I tried making a startup script that would move it back to origin then run the felling script again. I added it to the setup function I mentioned so all you have to do is download the setup file and run it the start "trees". The problem is that even after rebooting, it is stuck. So the script gets the location from gps, then tries to move back to the origin. But it can't move and just reboots over and over again.