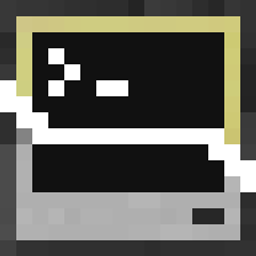
Port `fs.find` to Lua/CraftOS
SquidDev opened this issue ยท 1 comments
Currently fs.find
is implemented in Java, inside the main filesystem implementation.
This isn't really a problem most of the time. However, if someone wants to replace the fs
API (for instance, adding file permissions or custom mounts), then fs.find
no longer has the correct behaviour, and so must be reimplemented.
Given the current implementation only uses the "public" FS functions (list
, isDir
), it should be fairly easy to port this to Lua. It feels like it would also be a good time to make some improvements to the implementation:
- Add support for single-character wildcards (
?
). - Be more efficient in how we visit files.
fs.find("*")
still walks the entire file tree, while it should only need to be anfs.list
.
There's probably more improvements we could make here ([abc]
, [a-c]
, **
), but those can probably wait for later.
We'll want to flesh out the tests for fs.find before starting this - I've definitely some uncertainties about how paths are sanitised.
I've already made this for just this reason. Feel free to integrate it in the ROM if you have the time.