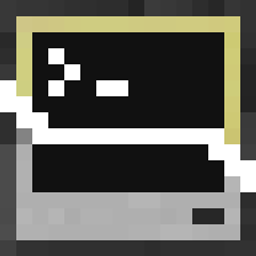
Support Lua callbacks as parameters to @LuaFunction methods
kwyntes opened this issue · 4 comments
To illustrate:
// currently not supported
@LuaFunction
public void callMe(ILuaFunction callback) {
var result = callback.call(new ObjectArguments("Java called, they want a return value back")).getResult();
// ... (use result in some way) ...
}
Although this is a feature request, I guess this is also more of a technical inquiry as to why this currently isn't supported. I suppose the intended way to interop with the computer from the Java API is to queue events that are captured by os.pullEvent
, and let the Lua script "return" values by calling a @LuaFunction
Java method to pass stuff back to the Java code. This does not make for nice APIs on either end.
Does Lua's execution model not allow callbacks like this (due to the way coroutines work or something)?
Can you show quite what you're trying to do? CC does a lot of async code, and nothing ends up looking quite like this.
So I'm trying to interface with external code (in this case, JavaScript through the V8 engine), and that code may want to interact with the computer to call peripheral methods or draw to the screen or something else.
For drawing to the screen at least, there's no need for getting values back from Lua, but for peripheral methods there is.
I could implement this by writing an event loop in Lua that responds to events generated by the external code, using something like this:
local module = externInterop.loadJSModule("module.js")
module.myMethod()
while true do
local _, name, params = os.pullEvent("ext_peripheral_call")
module.acceptPeripheralCallResult(peripheral.call(name, table.unpack(params)))
end
// the imported javascript module
let peripheralCallResultListener;
export function acceptPeripheralCallResult(result: any) {
peripheralCallResultListener(result);
}
export function myMethod() {
peripheralCallResultListener = (result) => { /* do something with result here */ }
queueLuaEvent("ext_peripheral_call", "chest_0", ["list"]);
}
I would much prefer if it were possible to simply supply a table containing the functions that can be used in the external code like this:
local module = externInterop.loadJSModule("module.js")
module.myMethod({
peripheralCall = function(name, params) return peripheral.call(name, table.unpack(params)) end
})
// the imported javascript module
export async function myMethod(luaApi: { peripheralCall: async (name: string, ...params: any[]) => any }) {
const result = await luaApi.peripheralCall("chest_0", "list");
// do something with result here
}
I think it's clear why the second version is a lot nicer than first. It might just be me being not smart enough to come up with anything cleaner than the first method for the current implementation though. It would still be nice to not have to write the event handling code in Lua.
which means you can only run these callbacks inside the body of a peripheral/API method.
That would be the case in my above example right? (just checking if I understand correctly)
So there's no technical reason as-such, but any usage of a callback function is both limited and awkward, so it has never felt worth exposing.
-
Lua is single threaded (and only runs in the CC thread-pool), which means you can only run these callbacks inside the body of a peripheral/API method. This means there's very few cases where you can do something useful with the callback.
-
Lua functions can yield at any point, so you won't be able to call
MethodResult.getResult()
directly. Instead, we'd need to either:- Add a
CompletableFuture.thenApplyAsync
toMethodResult
, so you supply a continuation that is run once the method has finished executing. More annoying to implement, but easier to consume. - Add a
MethodResult.call(ILuaFunction, ILuaCallback continuation, Object... args)
function. More annoying to consume, but easier to implement.
That said, I generally recommend against
ILuaCallback
/LuaTask
(for instance, preferring@LuaFunction(mainThread=true)
overILuaContext.executeMainThreadTask
). If/when we implement persistence, all of these functions need to be serialisable too, which is very awkward to do! - Add a
I suppose the intended way to interop with the computer from the Java API is to queue events that are captured by
os.pullEvent
, and let the Lua script "return" values by calling a@LuaFunction
Java method to pass stuff back to the Java code.
Can you show quite what you're trying to do? CC does a lot of async code, and nothing ends up looking quite like this.
Ahh, thanks for the additional context!
Oh, trying to map Lua and JS functions on to each other is tricky — promises are very different to CC's event queue. It might be worth getting pullEvent
working first if you haven't already? That one is available via the Java API right now (MethodResult.pullEvent
), but I think it's implementation and behaviour are not obvious to me (e.g. what should await Promise.all([os.pullEvent(), os.pullEvent()])
do?).
My suspicion here is that Lua functions called from JS will have to be called in a fresh coroutine (so they can be run in parallel, and generally have the correct promise semantics). At that point your best bet is probably to write a Lua module that wraps the underlying peripheral — it can provide a friendly callback-based API, and then do the conversion behind the scenes.
which means you can only run these callbacks inside the body of a peripheral/API method.
That would be the case in my above example right? (just checking if I understand correctly)
That's probably a little dependent on where you're running the JS code. I'd probably recommend running it on another thread, that way long-running JS code won't block other computers from working.
That's probably a little dependent on where you're running the JS code. I'd probably recommend running it on another thread, that way long-running JS code won't block other computers from working.
I think what he want here is basically CC:T but using another language (JS).
(e.g. what should await Promise.all([os.pullEvent(), os.pullEvent()]) do?).
I have no idea how to do that in JS either, so the best solution will be event callbacks but not directly yielding for events.
Actually technically, it should returns two same events in an array I think, since the pullEvent promises starts at same time.
As a reference, you can checkout https://github.com/zyxkad/cc/blob/master/coroutinex.lua, which I wrote a JS async like API in Lua.