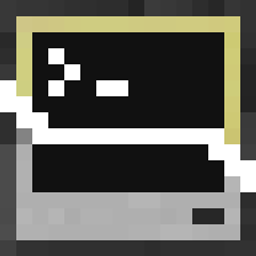
Error in error handling when calling recursive function
Kolterdyx opened this issue ยท 3 comments
Mod version: 1.96.0
Minecraft version: 1.16.5
Forge version: 36.1.25
I am trying to write some code to format a file so it can be printed by the printer peripheral without losing content (fitting the line length to the margins and stuff).
To do so I have written a recursive function, but upon execution, I get an error that just says error in error handling
This is the whole program:
filename = arg[1]
title = arg[2] or filename
file = fs.open(filename, 'r')
printer = peripheral.find("printer")
------- TEXT PROCESSING ----------
limit = 26
buffer_size = 10
serial = textutils.serialise
-- Adds all lines to a table and counts them
lines = {}
n = 0
while true do
a = file.readLine()
if a == nil then break end
n = n+1
table.insert(lines, a)
end
file.close()
-- Creates a buffer so we can process the text in chunks and not all at the same time
buffered_lines = {}
buffer = {}
for i,line in pairs(lines) do
if i%buffer_size==0 then
table.insert(buffered_lines, buffer)
buffer = {}
end
table.insert(buffer, line)
end
table.insert(buffered_lines, buffer)
number_of_lines = n -- lazy renaming of the number of lines
-- function to split a string into words
function split(str)
local result = {}
for word in string.gmatch(str, "[^%s]+") do
table.insert(result, word)
end
return result
end
-- recursive function to split a line until all the "sublines" are shorter than the limit
function split_line(line)
local result = {}
local to_next_line = false
local len = 0
local result_line = {}
local next_line = {}
for _,word in pairs(line) do
len = len + #word + 1
-- I check if adding another word to the line makes it too long or if we are creating a new line
if len > limit or to_next_line then
table.insert(next_line, word) -- if so, I add it and all the following words to the next line
to_next_line = true
else
table.insert(result_line, word) -- if not, I add it to the resulting line
end
end
table.insert(result, result_line) -- I add the resulting line to the result table
if next_line and #next_line > 0 and next_line ~= {} then -- I do not trust lua so I check everything just in case
for k,v in pairs(split_line(next_line)) do -- If the new line I created is longer than the limit, it should be split again, hence the recursion
table.insert(result, v)
end
end
return result -- pretty self-explanatory
end
final_buffer = {}
for _,buffer in pairs(buffered_lines) do
-- splits all the lines into words
split_lines = {}
for k,v in pairs(buffer) do
table.insert(split_lines, split(v))
end
-- limits the lines to a certain length
lim_lines = {}
for _,sp_line in pairs(split_lines) do
for _,line in pairs(split_line(sp_line)) do
table.insert(lim_lines, line)
end
end
-- rejoins the words together
text = {}
for _,line in pairs(lim_lines) do
local result_line = ""
for _,word in pairs(line) do
result_line = result_line..word.." "
end
result_line = result_line:sub(1, -2)
table.insert(text, result_line)
end
-- Saves the processed buffer
table.insert(final_buffer, text)
end
processed_text = {}
-- Moves everything from the buffer to a 1D table
for _,buffer in pairs(final_buffer) do
for _,line in pairs(buffer) do
table.insert(processed_text, line)
end
end
-- Separates the processed text into different pages (like buffers, but page-sized)
paged_text = {}
page = {}
for i,line in pairs(processed_text) do
if i%21 == 0 then
table.insert(paged_text, page)
page = {}
end
table.insert(page, line)
end
------- END OF TEXT PROCESSING ----------
function add(line, pos)
printer.setCursorPos(1, pos)
print(line, pos)
printer.write(line)
end
This is not about my code, it's about the error it throws. It says error in error handling
, which sounds like an exception implemented in the mod for when it isn't able to handle an error thrown either by Lua or by it's intepreter. I have tried recursively iterating through small tables, but when they are too big it either throws a StackOverflowError or the error in error handling
thing.
TL;DR
Recursive functions throw error in error handling
when they get too deep instead of a proper error.
Code needed to replicate:
function recursive_function()
recursive_function()
end
recursive_function()
@SquidDev I am using Mildly Better Shell