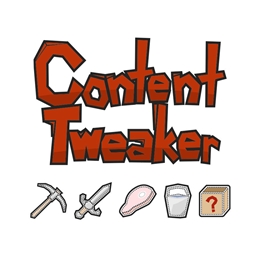
ItemDestroyedBlock function and AxisAlignedBB trouble
Kazerima opened this issue ยท 1 comments
I'm trying to create a test item that replaces a stone block that's broken with the tool with a dirt block instead, but the item doesn't place the dirt to replace it. In addition, I'm trying to make a 1/8 height block but it's also not registering properly. The documentation for ItemDestroyedBlock on the CraftTweaker page is not very clear, what am I doing wrong?
Also, is there a script command for CraftTweaker to give the player an item when 'foo' item is used? The best I can find maybe trying to call a command block command to give a specific player an item, but that doesn't really work for general case things does it? Is there a way to get the player's username if that's the method I have to use?
My code for the item is:
#loader contenttweaker
import mods.contenttweaker.VanillaFactory;
import mods.contenttweaker.ActionResult;
import mods.contenttweaker.BlockState;
import mods.contenttweaker.IItemDestroyedBlock;
var item2 = VanillaFactory.createItem("block changer");
item2.maxStackSize = 1;
item2.maxDamage = 1;
item2.itemDestroyedBlock = function(stack, world, blockState, pos, player) {
if (blockState == <block:minecraft:stone>) {
world.setBlockState(<block:minecraft:dirt>, pos);
stack.damage(1, player);
return true;
}
return false;
};
item2.register();
My code for the block is:
#loader contenttweaker
import mods.contenttweaker.VanillaFactory;
import mods.contenttweaker.Block;
import crafttweaker.item.IItemStack;
import mods.contenttweaker.AxisAlignedBB;
var ashBlock = VanillaFactory.createBlock("ash_block");
ashBlock.setLightOpacity(0);
ashBlock.setLightValue(0);
ashBlock.setToolClass("shovel");
ashBlock.setToolLevel(1);
ashAx = AxisAlignedBB.create(0, 0, 0, 1, 0.125, 1);
ashBlock.axisAlignedBB = ashAx;
ashBlock.register();
Sorry, this answer will be a little longer than my average answer length:
For your replacing issue:
Simple: It does place the dirt. You don't see it though, since the event is called before the block is removed from the world, so the dirt block is removed directly after it has been placed. Try placing the block at some offset to see what I'm talking about.
The block is not registered correctly because you have syntax errors:
- createBlock requires a blockType which you didn't provide
- You are assigning to ashAx without ever declaring it. Also, you don't need that variable because you only use it once.
Also, you need to change the render model in order for the block to then be rendered correctly, it defaults to full block rendering.
In case you need a little reference:
var ashBlock = VanillaFactory.createBlock("ash_block", <blockMaterial:wood>);
ashBlock.setLightOpacity(0);
ashBlock.setLightValue(0);
ashBlock.setToolClass("shovel");
ashBlock.setToolLevel(1);
ashBlock.axisAlignedBB = AxisAlignedBB.create(0, 0, 0, 1, 0.5, 1);
ashBlock.register();
Now we also need to change resources/contenttweaker/blockstates/ash_block.json
Here's what I used:
{
"forge_marker": 1,
"defaults": {
"textures": {
"bottom": "blocks/planks_birch",
"top": "blocks/cobblestone",
"side": "blocks/stone"
},
"model": "half_slab",
"uvlock": true,
"transform": "forge:default-block"
},
"variants": {
"normal": [{}],
"inventory": [{}]
}
}
Does it work? Kinda, there are still rendering issues when placed on walls, I just wanted to show an example, I'm not too good with graphics myself, that's why I just used Vanilla models/resources ^^