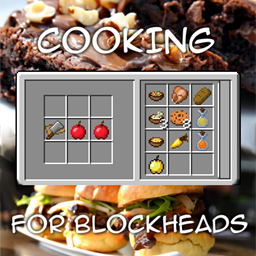
How do I make my mod use your Java API?
StupidRepo opened this issue ยท 8 comments
How do I exactly make my Food item (Chocolate) be crafted with the Cooking Table in this mod? I will use the Java API but there is no tutorial on how the API works. Please can you give me some example code on how to do these please? Thanks in advance, lol.
Edit: What should I put in my build.gradle
to link this API/mod with my mod?
Ok so I linked it in the Gradle thingy but idk what to do in my code.
Let's start with the important stuff at the top here.
My build.gradle
(full)
buildscript {
repositories {
maven { url = 'https://maven.minecraftforge.net' }
mavenCentral()
}
dependencies {
classpath group: 'net.minecraftforge.gradle', name: 'ForgeGradle', version: '4.1.+', changing: true
}
}
apply plugin: 'net.minecraftforge.gradle'
apply plugin: 'eclipse'
version = '1.0'
group = 'com.yourname.modid'
archivesBaseName = 'modid'
sourceCompatibility = targetCompatibility = compileJava.sourceCompatibility = compileJava.targetCompatibility = '1.8'
minecraft {
mappings channel: 'snapshot', version: '20201028-1.16.3'
runs {
client {
workingDirectory project.file('run')
property 'forge.logging.markers', 'REGISTRIES'
property 'forge.logging.console.level', 'debug'
mods {
examplemod {
source sourceSets.main
}
}
}
server {
workingDirectory project.file('run')
property 'forge.logging.markers', 'REGISTRIES'
property 'forge.logging.console.level', 'debug'
mods {
examplemod {
source sourceSets.main
}
}
}
}
}
dependencies {
minecraft 'net.minecraftforge:forge:1.16.5-36.1.0'
compile fg.deobf("curse.maven:cookingforblockheads-231484:3330395")
}
repositories {
maven {
url "https://minecraft.curseforge.com/api/maven/"
}
maven {
name = "Progwml6 maven"
url = "https://dvs1.progwml6.com/files/maven/"
}
maven {
url "https://www.cursemaven.com"
}
flatDir {
dirs "run/obfmods"
}
}
My code (full)
package epic.mods.foodies;
import net.minecraftforge.fml.event.server.FMLServerStartingEvent;
import net.minecraftforge.fml.event.lifecycle.FMLCommonSetupEvent;
import net.minecraftforge.fml.event.lifecycle.FMLClientSetupEvent;
import net.minecraftforge.fml.common.Mod;
import net.minecraftforge.eventbus.api.SubscribeEvent;
import net.minecraftforge.event.world.BiomeLoadingEvent;
import net.minecraftforge.api.distmarker.OnlyIn;
import net.minecraftforge.api.distmarker.Dist;
import net.minecraftforge.fml.event.lifecycle.InterModProcessEvent;
import net.minecraft.item.ItemStack;
import net.minecraftforge.client.model.obj.MaterialLibrary;
@Mod.EventBusSubscriber(bus = Mod.EventBusSubscriber.Bus.MOD)
public class TestFunky {
public TestFunky() {
ItemStack inp_stick = new ItemStack(Material.STICK);
ItemStack out_stick = new ItemStack(Material.ANVIL);
addOvenRecipe(inp_stick, out_stick); // ERRORS HERE, WHY?
}
@SubscribeEvent
public static void init(FMLCommonSetupEvent event) {
new TestFunky();
}
@SubscribeEvent
public static void serverLoad(FMLServerStartingEvent event) {
}
@OnlyIn(Dist.CLIENT)
@SubscribeEvent
public static void clientLoad(FMLClientSetupEvent event) {
}
@Mod.EventBusSubscriber
private static class ForgeBusEvents {
// Example Forge bus event registration
@SubscribeEvent
public static void addFeatureToBiomes(BiomeLoadingEvent event) {
}
}
}
So, how do I use the api now?
I get an error that says crap like "error: cannot find symbol" for this bit of code: import net.blay09.mods.cookingforblockheads.api;
IMPORTANT
I want to add my mod item, Chocolate to the Cooking Table (said Chocolate requires 3 wheat, 1 cocoa bean, 2 sugar and 3 milk buckets, just like a cake recipe.
W = Wheat
C = Cocoa Bean(s)
S = Sugar
M = Milk Bucket(s)
[ M | M | M ]
[ S | C | S ]
[ W | W | W ]
See https://www.cursemaven.com/ for how to use mods from Curse as dependencies.
But also the use of Java API seems overkill here, all you need is to include a json file like this in your mod:
Where do I put the JSON file? Could you give me a tutorial on this. I would use the IMC API but I don't know how to call IMC functions and from what I've seen, your IMC API has no @Mod(modid = "receiver", name = ":)", version = "1.0.0")
so I can't call the functions. Unless I am thick and the modid
is cookingforblockheads
?
But also the use of Java API seems overkill here, all you need is to include a json file like this in your mod:
https://github.com/blay09/CookingForBlockheads/tree/1.16.x/src/main/resources/data/cookingforblockheads/cookingforblockheads/compat
WAIT so your saying the I need some compat folder? If so, what do I do for that then and what do I put in the JSON file?
@blay09:
all you need is to include a json file like this in your mod:
What do I put in said JSON file and where do I put said compat folder?