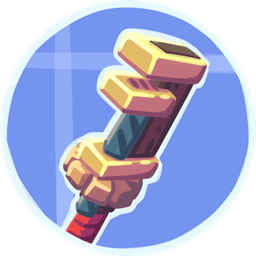
[Build Error] Unsupported type provided to method 'validBlocks' in AllTileEntities.java
ugudango opened this issue ยท 2 comments
After I run ./gradlew build
I get the following error:
error: method validBlocks in class TileEntityBuilder<T,P> cannot be applied to given types;
.validBlocks(AllBlocks.DYED_VALVE_HANDLES)
^
required: NonNullSupplier<? extends Block>[]
found: BlockEntry<?>[]
reason: varargs mismatch; BlockEntry<?>[] cannot be converted to NonNullSupplier<? extends Block>
where T,P are type-variables:
T extends TileEntity declared in class TileEntityBuilder
P extends Object declared in class TileEntityBuilder
I have looked into the issue and it seems like validBlocks
is a variadic function that takes in more non-null Block
based classes:
@SafeVarargs
public final TileEntityBuilder<T, P> validBlocks(NonNullSupplier<? extends Block>... blocks) {
Arrays.stream(blocks).forEach(this::validBlock);
return this;
}
In AllTileEntities
, however, within the builder calls, AllBlocks.DYED_VALVE_HANDLES
is provided, which is an array of a Block
based class:
public static final BlockEntry<?>[] DYED_VALVE_HANDLES = new BlockEntry<?>[DyeColor.values().length];
Under normal circumstances, this should, however, work - passing arrays to variadic functions is intended behaviour in Java.
I have made a patch, but since I'm not a Java dev mainly, and I've never made any mods, I consider it to be quite terrible.
The build works and the mod seems to have no issues. Then again, I might just use the wrong prerequisites and have that cause a problem.
So what I came up with was specializing the DYED_VALVE_HANDLES
in AllBlocks.java
:
- public static final BlockEntry<?>[] DYED_VALVE_HANDLES = new BlockEntry<?>[DyeColor.values().length];
+ public static final BlockEntry<ValveHandleBlock>[] DYED_VALVE_HANDLES = new BlockEntry[DyeColor.values().length];
Besides this, I've also "split" the builder chain, which also seemed to work. This is, however, way messier.
In AllTileEntities.java
- line 194
- public static final TileEntityEntry<HandCrankTileEntity> HAND_CRANK = Create.registrate()
- .tileEntity("hand_crank", HandCrankTileEntity::new)
- .validBlocks(AllBlocks.HAND_CRANK, AllBlocks.COPPER_VALVE_HANDLE)
- .validBlocks(AllBlocks.DYED_VALVE_HANDLES)
- .renderer(() -> HandCrankRenderer::new)
- .register();
+ public static TileEntityEntry<HandCrankTileEntity> HAND_CRANK;
+
+ static {
+ TileEntityBuilder<HandCrankTileEntity, CreateRegistrate> PARTIAL = Create.registrate()
+ .tileEntity("hand_crank", HandCrankTileEntity::new)
+ .validBlocks(AllBlocks.HAND_CRANK, AllBlocks.COPPER_VALVE_HANDLE);
+
+ for (BlockEntry<?> B : AllBlocks.DYED_VALVE_HANDLES) {
+ PARTIAL = PARTIAL.validBlocks(B);
+ }
+
+ AllTileEntities.HAND_CRANK = PARTIAL
+ .renderer(() -> HandCrankRenderer::new)
+ .register();
+ }