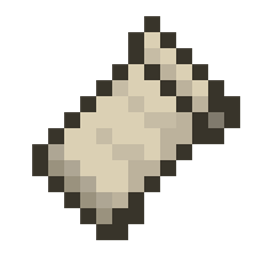
I can't give the cakes my properties
Miros77 opened this issue · 10 comments
error: The method tryEat(WorldAccess, BlockPos, BlockState, PlayerEntity) of type ModCakeBlock must override or implement a supertype method
I would recommend using a custom mixin for this if you want to change the vanilla cake. This is not something that fabric api is likely to support any time soon. It should be as easy as targeting the tryEat method in the CakeBlock.class
and modfying the constants for food and saturation using a @ModifyConstant
mixin.
Your second message is because your child class is not implementing all of the methods that it's required to implement by the parent. Going back to what it says "ModCakeBlock must [define the method] tryEat(WorldAccess, BlockPos, BlockState, PlayerEntity)" because it inherits from CakeBlock. https://www.tutorialspoint.com/java/java_inheritance.htm
I would recommend using a custom mixin for this if you want to change the vanilla cake. This is not something that fabric api is likely to support any time soon. It should be as easy as targeting the tryEat method in the
CakeBlock.class
and modfying the constants for food and saturation using a@ModifyConstant
mixin.Your second message is because your child class is not implementing all of the methods that it's required to implement by the parent. Going back to what it says "ModCakeBlock must [define the method] tryEat(WorldAccess, BlockPos, BlockState, PlayerEntity)" because it inherits from CakeBlock. https://www.tutorialspoint.com/java/java_inheritance.htm
here's the code that doesn't work
package com.miros77.rbp.registry.all_cake;
import net.minecraft.block.CakeBlock;
import net.minecraft.block.BlockState;
import net.minecraft.entity.player.PlayerEntity;
import net.minecraft.item.ItemStack;
import net.minecraft.stat.Stats;
import net.minecraft.util.ActionResult;
import net.minecraft.util.Hand;
import net.minecraft.util.hit.BlockHitResult;
import net.minecraft.util.math.BlockPos;
import net.minecraft.world.World;
import net.minecraft.world.WorldAccess;
public class ModCakeBlock extends CakeBlock {
public ModCakeBlock(Settings settings) {
super(settings);
}
@OverRide
public ActionResult onUse(BlockState state, World world, BlockPos pos, PlayerEntity player, Hand hand, BlockHitResult hit) {
if (world.isClient) {
ItemStack itemStack = player.getStackInHand(hand);
if (this.tryEat(world, pos, state, player).isAccepted()) {
return ActionResult.SUCCESS;
}
if (itemStack.isEmpty()) {
return ActionResult.CONSUME;
}
}
return this.tryEat(world, pos, state, player);
}
@OverRide
public ActionResult tryEat(WorldAccess world, BlockPos pos, BlockState state, PlayerEntity player) {
if (!player.canConsume(false)) {
return ActionResult.PASS;
} else {
player.incrementStat(Stats.EAT_CAKE_SLICE);
player.getHungerManager().add(2, 0.1F);
int i = (Integer)state.get(BITES);
if (i < 6) {
world.setBlockState(pos, (BlockState)state.with(BITES, i + 1), 3);
} else {
world.removeBlock(pos, false);
}
return ActionResult.SUCCESS;
}
}
}
I would recommend using a custom mixin for this if you want to change the vanilla cake. This is not something that fabric api is likely to support any time soon. It should be as easy as targeting the tryEat method in the
CakeBlock.class
and modfying the constants for food and saturation using a@ModifyConstant
mixin.
I would recommend using a custom mixin for this if you want to change the vanilla cake. This is not something that fabric api is likely to support any time soon. It should be as easy as targeting the tryEat method in the CakeBlock.class and modfying the constants for food and saturation using a @ModifyConstant mixin. - when will it be done
public class ChokoladeCakeBlock extends CakeBlock {
public ChokoladeCakeBlock() {
super(FabricBlockSettings.of(Material.CAKE));
}
public ActionResult onUse(BlockState state, World world, BlockPos pos, PlayerEntity player, Hand hand, BlockHitResult hit) {
if (world.isClient) {
ItemStack itemStack = player.getStackInHand(hand);
if (this.tryEat(world, pos, state, player).isAccepted()) {
return ActionResult.SUCCESS;
}
if (itemStack.isEmpty()) {
return ActionResult.CONSUME;
}
}
return this.tryEat(world, pos, state, player);
}
public ActionResult tryEat(WorldAccess world, BlockPos pos, BlockState state, PlayerEntity player) {
if (!player.canConsume(false)) {
return ActionResult.PASS;
} else {
player.incrementStat(Stats.EAT_CAKE_SLICE);
player.getHungerManager().add(7, 0.5F);
int i = (Integer)state.get(BITES);
if (i < 6) {
world.setBlockState(pos, (BlockState)state.with(BITES, i + 1), 3);
} else {
world.removeBlock(pos, false);
}
return ActionResult.SUCCESS;
}
}
}
Is this your extended cake or the vanilla one? (lmao I should have just read)
For the extended cake, you would add the property via appendProperties