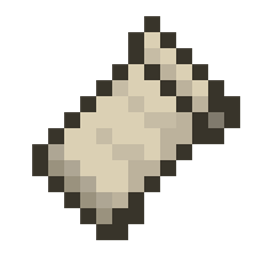
Clientside chunk cache grows unbounded
parzivail opened this issue ยท 4 comments
Tested on 1.18.2, Loader 0.14.4
Continuously generating chunks allows the Set<WorldChunk> loadedChunks
field, accessed by the interface net.fabricmc.fabric.impl.event.lifecycle.LoadedChunksCache
(in the chart as lcc
) and mixed into ClientWorld
through net.fabricmc.fabric.mixin.event.lifecycle.client.ClientWorldMixin
, to grow unbounded on the client. On the server chunks are correctly removed via the LoadedChunksCache
interface method fabric_markUnloaded
, but this does not seem to happen on the client. Over time this completely saturates the heap.
X value on all charts is time in ticks. Chunks are continuously being generated by automatically teleporting the player periodically.
Memory heap usage vs vanilla and LCC cache size on client and server
Memory usage vs client LCC cache size after manually periodically calling clear
on the set
Memory usage vs client LCC cache size with a more aggressive manual set clearing
There is a known issue if you have a mod that changes the chunk(un)loading in a certain way, see #1823
There are also repeated reports of problems with this code that are really other mods trying to do things on multiple or wrong threads. Something that minecraft and therefore fabric doesn't support.
Beyond that you will need to figure out how to reproduce the issue, e.g.
- which mod is causing the problem - does it occur with only fabric api installed?
- what mods do you have that manipulate chunk loading?
- what activities causes the problem - last time this was a problem it was related to a large number of teleports #1819
- maybe there are warnings or errors in your log that might hint at something wrong?
- etc.
A minimal code sample that can reproduce the issue in a timely manner, via teleporting the player in a spiral after no chunk tasks have been pending for at least 30 ticks (assuming view distance is 32 chunks):
This code sample was used to produce the graphs in the original post.
public final class ContinuousChunkGen implements ModInitializer
{
private static int i = 0;
private static final int MIN_TIME_INTERVAL = 30;
private static int timer = MIN_TIME_INTERVAL;
@Override
public void onInitialize()
{
ServerTickEvents.START_WORLD_TICK.register(ContinuousChunkGen::onWorldTick);
}
private static void onWorldTick(ServerWorld world)
{
var players = world.getPlayers();
if (players.isEmpty())
return;
var player = players.get(0);
if (world.getChunkManager().getPendingTasks() != 0)
timer = MIN_TIME_INTERVAL;
timer--;
if (timer > 0)
return;
timer = MIN_TIME_INTERVAL;
i++;
var t1 = 10 * (i / (100 * Math.PI));
var x = 512 * (t1 * Math.cos(t1) / (2 * Math.PI));
var z = 512 * (t1 * Math.sin(t1) / (2 * Math.PI));
player.teleport(x, 100, z);
}
}
- There are no mods installed, i.e. this issue is caused by FAPI
- No errors or warnings are reported to the log, except the crash when nothing more can be allocated on the heap
- Issue is reproducible through any means of generating chunks in vanilla, by continuously walking, teleporting, etc.
Symptoms and setup overlap with those described in #1819, but can additionally be reproduced without teleporting
Looks to me like the fix for #1819 was never applied to the 1.18x branch?
Maybe you can create a temporary fix for yourself to confirm that is problem?