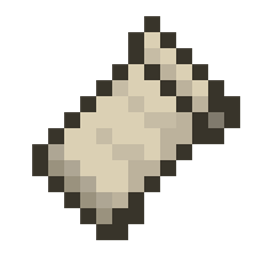
Rendering outlines for specific blocks.
anarchy1337x opened this issue ยท 1 comments
Error
FATAL ERROR in native method: Thread[Render thread,10,main]: No context is current or a function that is not available in the current context was called. The JVM will abort execution.
at org.lwjgl.opengl.GL11.glPushMatrix(Native Method)
at me.anarchy.demo.demo.client.demoClient.lambda$onInitializeClient$0(demoClient.java:75)
at me.anarchy.demo.demo.client.demoClient$$Lambda$3772/0x0000000801535068.beforeBlockOutline(Unknown Source)
at net.minecraft.client.render.WorldRenderer.handler$zhm000$beforeRenderOutline(WorldRenderer.java:3496)
at net.minecraft.client.render.WorldRenderer.render(WorldRenderer.java:1168)
at net.minecraft.client.render.GameRenderer.renderWorld(GameRenderer.java:1024)
at net.minecraft.client.render.GameRenderer.render(GameRenderer.java:833)
at net.minecraft.client.MinecraftClient.render(MinecraftClient.java:1102)
at net.minecraft.client.MinecraftClient.run(MinecraftClient.java:752)
at net.minecraft.client.main.Main.main(Main.java:220)
at net.minecraft.client.main.Main.main(Main.java:56)
at java.lang.invoke.LambdaForm$DMH/0x0000000800e18000.invokeStaticInit([email protected]/LambdaForm$DMH)
at java.lang.invoke.LambdaForm$MH/0x0000000800c01800.invokeExact_MT([email protected]/LambdaForm$MH)
at net.fabricmc.loader.impl.game.minecraft.MinecraftGameProvider.launch(MinecraftGameProvider.java:461)
at net.fabricmc.loader.impl.launch.knot.Knot.launch(Knot.java:74)
at net.fabricmc.loader.impl.launch.knot.KnotClient.main(KnotClient.java:23)
at java.lang.invoke.LambdaForm$DMH/0x0000000800c01000.invokeStatic([email protected]/LambdaForm$DMH)
at java.lang.invoke.LambdaForm$MH/0x0000000800c01800.invokeExact_MT([email protected]/LambdaForm$MH)
at net.fabricmc.devlaunchinjector.Main.main(Main.java:86)l
Context
I'm trying to render outlines of specific blocks that are visible through walls, i've tried multiple times to use the raw opengl libraries, but i get the same issue everytime, no context specified. I've tried looking through the documentation, and various forge-alternatives. I've attempted to use pre-made libraries to render the boxes, it does it successfully but the offset is not at the block, and doesn't change no matter where i move.
I've been researching and apparently i need to use the tesselator and the built-in bufferbuilder, i'd love some guidance (and|or) clarification on what i should use.
Thanks!
GL11.glEnable(GL11.GL_BLEND);
GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA);
GL11.glEnable(GL11.GL_LINE_SMOOTH);
GL11.glLineWidth(2);
GL11.glDisable(GL11.GL_TEXTURE_2D);
GL11.glEnable(GL11.GL_CULL_FACE);
GL11.glDisable(GL11.GL_DEPTH_TEST);
double renderPosX = instance.gameRenderer.getCamera().getPos().getX();
double renderPosY = instance.gameRenderer.getCamera().getPos().getY();
double renderPosZ = instance.gameRenderer.getCamera().getPos().getZ();
GL11.glPushMatrix();
GL11.glTranslated(-renderPosX, -renderPosY, -renderPosZ);
GL11.glColor4f(0, 1, 0, 0.25F);
for(BlockEntity blockEntity : getBlockEntities()) {
assert instance.world != null;
Block block = instance.world.getBlockState(blockEntity.getPos()).getBlock();
if(block.getTranslationKey().endsWith("chest")) {
BlockPos blockPosition = blockEntity.getPos();
int x = blockPosition.getX();
int y = blockPosition.getX();
int z = blockPosition.getX();
Box box = new Box(x, y, z, x + 1, y + 1, z + 1);
RenderUtils.drawOutlinedBox(box, matrixStack);
}
}
GL11.glPopMatrix();
GL11.glEnable(GL11.GL_DEPTH_TEST);
GL11.glEnable(GL11.GL_TEXTURE_2D);
GL11.glDisable(GL11.GL_BLEND);
GL11.glDisable(GL11.GL_LINE_SMOOTH);
drawOutlinedBox
public static void drawOutlinedBox(Box bb, MatrixStack matrixStack) {
Matrix4f matrix = matrixStack.peek().getPositionMatrix();
Tessellator tessellator = RenderSystem.renderThreadTesselator();
BufferBuilder bufferBuilder = tessellator.getBuffer();
RenderSystem.setShader(GameRenderer::getPositionShader);
bufferBuilder.begin(VertexFormat.DrawMode.DEBUG_LINES, VertexFormats.POSITION);
bufferBuilder.vertex(matrix, (float)bb.minX, (float)bb.minY, (float)bb.minZ).next();
bufferBuilder.vertex(matrix, (float)bb.maxX, (float)bb.minY, (float)bb.minZ).next();
bufferBuilder.vertex(matrix, (float)bb.maxX, (float)bb.minY, (float)bb.minZ).next();
bufferBuilder.vertex(matrix, (float)bb.maxX, (float)bb.minY, (float)bb.maxZ).next();
bufferBuilder.vertex(matrix, (float)bb.maxX, (float)bb.minY, (float)bb.maxZ).next();
bufferBuilder.vertex(matrix, (float)bb.minX, (float)bb.minY, (float)bb.maxZ).next();
bufferBuilder.vertex(matrix, (float)bb.minX, (float)bb.minY, (float)bb.maxZ).next();
bufferBuilder.vertex(matrix, (float)bb.minX, (float)bb.minY, (float)bb.minZ).next();
bufferBuilder.vertex(matrix, (float)bb.minX, (float)bb.minY, (float)bb.minZ).next();
bufferBuilder.vertex(matrix, (float)bb.minX, (float)bb.maxY, (float)bb.minZ).next();
bufferBuilder.vertex(matrix, (float)bb.maxX, (float)bb.minY, (float)bb.minZ).next();
bufferBuilder.vertex(matrix, (float)bb.maxX, (float)bb.maxY, (float)bb.minZ).next();
bufferBuilder.vertex(matrix, (float)bb.maxX, (float)bb.minY, (float)bb.maxZ).next();
bufferBuilder.vertex(matrix, (float)bb.maxX, (float)bb.maxY, (float)bb.maxZ).next();
bufferBuilder.vertex(matrix, (float)bb.minX, (float)bb.minY, (float)bb.maxZ).next();
bufferBuilder.vertex(matrix, (float)bb.minX, (float)bb.maxY, (float)bb.maxZ).next();
bufferBuilder.vertex(matrix, (float)bb.minX, (float)bb.maxY, (float)bb.minZ).next();
bufferBuilder.vertex(matrix, (float)bb.maxX, (float)bb.maxY, (float)bb.minZ).next();
bufferBuilder.vertex(matrix, (float)bb.maxX, (float)bb.maxY, (float)bb.minZ).next();
bufferBuilder.vertex(matrix, (float)bb.maxX, (float)bb.maxY, (float)bb.maxZ).next();
bufferBuilder.vertex(matrix, (float)bb.maxX, (float)bb.maxY, (float)bb.maxZ).next();
bufferBuilder.vertex(matrix, (float)bb.minX, (float)bb.maxY, (float)bb.maxZ).next();
bufferBuilder.vertex(matrix, (float)bb.minX, (float)bb.maxY, (float)bb.maxZ).next();
bufferBuilder.vertex(matrix, (float)bb.minX, (float)bb.maxY, (float)bb.minZ).next();
tessellator.draw();
}
๐ We use the issue tracker exclusively for final bug reports and feature requests. However, this issue appears to be better suited for either a discussion thread, or a message on our discord server. Please post your request on one of these, and the conversation can continue there.