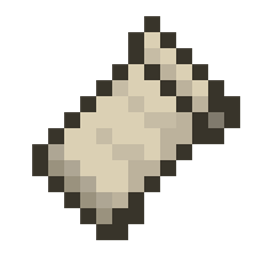
FabricBlockSettings.copyOf(Block.Settings) can mutate argument
reoseah opened this issue ยท 1 comments
FabricBlockSettings#copyOf(Block.Settings)
returns a wrapper of an argument. Calling methods such as 'hardness(...)` on this "copy" changes original, while it's expected to only change the copy.
public static FabricBlockSettings copyOf(Block.Settings settings) {
return new FabricBlockSettings(settings);
}
protected FabricBlockSettings(final Block.Settings delegate) {
this.delegate = delegate;
}
Instead, FabricBlockSettings#copyOf(Block.Settings)
should make a deep copy of an argument and then wrap it into FabricBlockSettings
. Method FabricBlockSettings#copy(Block)
, for example, calls Block.Settings#copy
, which does make a deep copy:
public static Block.Settings copy(Block source) {
Block.Settings settings = new Block.Settings(source.material, source.materialColor);
settings.material = source.material;
settings.hardness = source.hardness;
settings.resistance = source.resistance;
settings.collidable = source.collidable;
settings.randomTicks = source.randomTicks;
settings.luminance = source.lightLevel;
settings.materialColor = source.materialColor;
settings.soundGroup = source.soundGroup;
settings.slipperiness = source.getSlipperiness();
settings.dynamicBounds = source.dynamicBounds;
return settings;
}
This has since been fixed in object builder api v1.