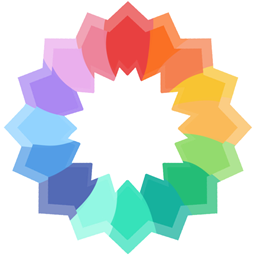
Add an interpolated temperature uniform
EtiTheSpirit opened this issue ยท 3 comments
I am designing a mock thermal vision shader. It currently uses a mixture of lighting values and block colors, but to further enhance the visuals, I implemented the temperature value provided by the mod. This addition works great, however for lack of any interpolation, there is a jarring and often extreme transition between color tones when changing biomes, a transition that becomes especially annoying around the borders of intermingled biomes.
This issue would be resolved if some form of interpolated temperature were provided. As far as the proposed timescale, I suggest using a uniform vec4
laid out such that the transition time is equal to (1 second, 2 seconds, 5 seconds, 10 seconds)
if possible.
I am not opposed to other implementations, the only real concrete interest is that a value is provided which is interpolated over a span of time around 5 seconds.
I will add now that I was able to achieve a temporary workaround using a buffer. It's not ideal, but will work.
uniform float temperature;
uniform float frameTime;
uniform int frameCounter;
layout(std430, binding=0) buffer temperatureBuffer {
vec2 lastTemperature;
};
#define BUFFER_INDEX_THIS_FRAME (frameCounter & 1)
#define BUFFER_INDEX_NEXT_FRAME ((frameCounter + 1) & 1)
// ...
void main() {
float lastTemp = lastTemperature[BUFFER_INDEX_THIS_FRAME];
float difference = temperature - lastTemp;
float effectiveDT = min(frameTime * 0.5, 1.0);
difference *= effectiveDT;
float realTemperature = lastTemp + difference;
lastTemperature[BUFFER_INDEX_NEXT_FRAME] = realTemperature;
}
Is this not possible using the custom uniform system?
I was not aware that this was a feature. Is this a new feature? I didn't see any mention of it (or perhaps I didn't notice it). I'll experiment with it later on, but if I can store a state (namely, store the previous value) then yes, it would be possible and I will close this issue.