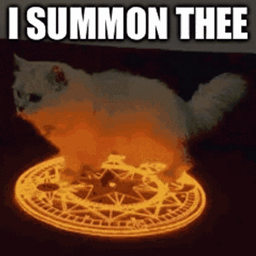
[Bug] not work when `replaceOut = Item.getEmpty()`
EvanHsieh0415 opened this issue ยท 11 comments
JEI Crafting: 1.2.0
KubeJS: 2101.7.1-build.181
Rhino: 2101.2.7-build.74
ServerEvents.recipes((event) => {
event.custom({
type: "jei_crafting:jei_crafting",
output: Item.of("golden_apple"),
ingredients: [
{
ingredient: {
item: "minecraft:apple",
},
count: 1,
},
{
ingredient: {
item: "minecraft:gold_block",
},
count: 8,
}
],
}).id("kubejs:golden_apple");
});
JeiCraftingEvents.itemCrafting("kubejs:golden_apple", (event) => {
// event.recipeOutput = Item.of("enchanted_golden_apple"); // works
event.recipeOutput = Item.getEmpty(); // doesn't work
})
btw
JeiCraftingEvents.itemCrafting("kubejs:golden_apple", (event) => {
console.log(event.recipeOutput); // 0 minecraft:air [net.minecraft.world.item.ItemStack]
event.recipeOutput = Item.getEmpty();
})
but recipe still output original item (minecraft:golden_apple)
Another thing I don't quite understand is why subsequent changes affect the previous output.
event.recipeOutput
is INTENTIONALLY empty and any modification will lead to a change to the final output. If you want to know the original output, try event.recipe.output()
to get the output from the recipe.
It is empty because the extra id is the RECIPE ID, so in any case you MUST know the output of the corresponding recipe already.
Can I choose not to play the sound during cancel? I want to play my customised sound.
Also, I found that I can't seem to get the number of times this synthesis was done, which can cause errors in the calculations.
To make you happy I already rewired shittons of code in the repo just to implement the KubeJS event compat, and I don't feel happy to make you happy anymore.
Crafting amount is not given because the output will be multiplied by the amount crafted, to be honest, I don't want to do anything that actually modifies the ItemStack
and DOES NOT reflect on the tooltip given, so I don't want to accept any of your suggestion anymore.
This is my mod and I designed it in a way I like, if you want to do anything you like then fork and make a PR to it.
You first asked a wrong question and then keep asking under this closed issue. Any time I open up the page and answer your question I feel like your old wrong question is just mocking me.
I'm sorry for making you feel this way, I was just trying to make the content I wanted with this mod and didn't realise that these requests would make you feel uncomfortable, I'm sorry.
I will try to use other methods to achieve this feature, I'm sorry for bothering you.
Here is the code I tried before:
/**
* @typedef TradeResult_
* @property {String} type
* @property {ItemStack_} tradeSign
* @property {(event: $JEICraftingCraftEvent_) => boolean} callback
*/
const TradeEntries = {
/** @param {number} exp @return {TradeResult_} */
experience: (exp) => ({
type: "experience",
tradeSign: TradeSignItemBuilder("experience", exp),
callback: (event) => {
event.player.addXP(exp * event.recipe.output().count);
event.player.level[
"playSound(net.minecraft.world.entity.Entity,net.minecraft.core.BlockPos,net.minecraft.sounds.SoundEvent,net.minecraft.sounds.SoundSource,float,float)"
](null, event.player.block.pos, "entity.experience_orb.pickup", "players", 1, 1);
return true;
},
}),
};
/**
* @param {string} type
* @param {Any} metadata
* @returns {$ItemStack_}
*/
const TradeSignItemBuilder = (type, metadata) => {
switch (type) {
case "experience":
return Item.of("name_tag")
.withLore(Text.translate("Add %s xp", metadata).gold().italic(false))
.withCustomName(Text.translate("Trade Sign - %s", type).darkGreen().italic(false));
default:
return Item.of("name_tag").withCustomName(Text.translate("Trade Sign - %s", type).darkGreen().italic(false));
}
};
/** @type {[ItemStack_[], TradeResult_][]} */
const TradeSignMapping = [
[["copper_block"], TradeEntries.experience(10)],
[["iron_block"], TradeEntries.experience(20)],
[["gold_block"], TradeEntries.experience(30)],
[["diamond_block"], TradeEntries.experience(40)],
[["emerald_block"], TradeEntries.experience(50)],
[["netherite_block"], TradeEntries.experience(60)],
];
TradeSignMapping.forEach(([item, data], index) => {
let { type, tradeSign, callback } = data;
let id = `kubejs:trade_sign/${type}/${index}`;
ServerEvents.recipes((event) => {
event
.custom({
type: "jei_crafting:jei_crafting",
output: tradeSign,
ingredients: item.map((item_) => {
let stack = Item.of(item_);
let ingredient = Ingredient.of(stack);
return {
ingredient: ingredient.itemIds.length > 1 ? { tag: stack.id } : { item: stack.id },
count: stack.count,
};
}),
})
.id(id);
});
JeiCraftingEvents.itemCrafting(id, (event) => {
if (callback(event)) {
event.cancel();
}
});
RecipeViewerEvents.addEntries("item", (event) => {
event.add(tradeSign);
});
});