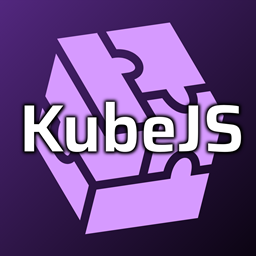
[Question] listen to unlocking of achievements
JairoQuispe opened this issue ยท 4 comments
If a player gets an achievement, for example "diamonds", is it possible listen to the unlocking of that event and run a script?
The event you're looking for is player.advancement
, which returns a PlayerAdvancementEventJS.
From there, you can get the advancement with event.advancement
as well as the player (or rather, entity) with event.entity
.
events.listen("player.advancement", function(event) {
if(event.advancement.getDisplayText() == "[Diamonds!]"){
event.player.give("minecraft:golden_apple");
}
});
How can I give items with enchantments and low durability ?
From the official KubeJS wiki, here are a couple of examples on how to get items:
// Pass item as a string. This uses the same syntax as /give commands would.
// If an oredict entry is supplied, the first item in that entry will be selected.
"minecraft:stone_sword" //Stone Sword
"minecraft:stone_sword 1 10" //Stone Sword with damage 10
"minecraft:apple 30" //30 Apples
"minecraft:compass {display:{Name:\"Spawn\"}}"
// Pass item as JS object. Additional keys are data, count, nbt, caps.
{item: "minecraft:stone_sword"}
{item: "minecraft:stone_sword", data: 10}
{item: "minecraft:apple", count: 30}
{item: "minecraft:compass", nbt: {display: {Name: "Spawn"}}}
// item.of() works with either of the above, but explicitly returns an ItemStackJS,
// so you can then chain functions to change the item further. (See ItemStackJS documentation for more info)
item.of("minecraft:stone_sword")
item.of("minecraft:stone_sword").data(10)
item.of("minecraft:apple").count(30)
item.of("minecraft:compass").name("Spawn")
item.of("minecraft:compass").name("Spawn").count(30)
in your case, i'd recommend either using javascript objects or item.of(), depending on what you prefer, so for example, if you wanted to give someone a stone sword with 10 damage and sharpness 1, you should be able to do this:
event.entity.give({
item: "minecraft:stone_sword",
data: 10,
nbt: {
Enchantments:[
{ id:"minecraft:sharpness", lvl:1 }
]
}
});