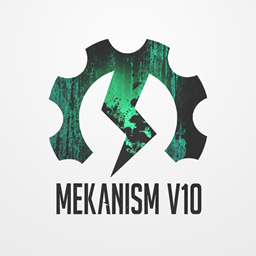
Adding Energized Smelter recipe
LucasBaizer opened this issue ยท 1 comments
Hi,
With the Mekanism API, there seems to be no way to add Energized Smelter recipes using the RecipeHelper class. I attempted to write a method to do this using Java's reflection:
private static void addEnergizedSmelterRecipe(ItemStack input, ItemStack output) {
try {
Class<?> itemInput = Class.forName("mekanism.common.recipe.input.ItemStackInput");
Class<?> itemOutput = Class.forName("mekanism.common.recipe.output.ItemStackOutput");
Class<?> recipeEnum = Class.forName("mekanism.common.recipe.RecipeHandler.Recipe");
Class<?> machineClass = Class.forName("mekanism.common.recipe.machines.MachineRecipe");
Class<?> recipeClass = Class.forName("mekanism.common.recipe.RecipeHandler");
Class<?> smeltingClass = Class.forName("mekanism.common.recipe.machines.SmeltingRecipe");
Constructor<?> inputConstructor = itemInput.getConstructor(ItemStack.class);
Constructor<?> outputContructor = itemOutput.getConstructor(ItemStack.class);
Constructor<?> smeltingConstructor = smeltingClass.getConstructor(itemInput, itemOutput);
Object inputInstance = inputConstructor.newInstance(input);
Object outputInstance = outputContructor.newInstance(output);
Object smeltingInstance = smeltingConstructor.newInstance(inputInstance, outputInstance);
Method m = recipeClass.getMethod("addRecipe", recipeEnum, machineClass);
m.invoke(null, recipeEnum.getField("ENERGIZED_SMELTER").get(null), smeltingInstance);
} catch (Exception e) {
System.err.println("[Mekanism] Error while adding recipe: " + e.getClass() + ": " + e.getMessage());
}
}
But this causes an exception, which is caught and printed out as:
[Mekanism] Error while adding recipe: class java.lang.ClassNotFoundException: mekanism.common.recipe.input.ItemStackInput
(P.S. my mod is forced to load after Mekanism)
What is the recommended way to add energized smelter recipes/way to fix this problem?
Okay, it took several hours, but I fixed the problem. I had the packages wrong for ItemStackInput/ItemStackOutput. Also, Class.forName("mekanism.common.recipe.RecipeHandler.Recipe")
is not the correct way to get a static subclass. It would be, rather, Class.forName("mekanism.common.recipe.RecipeHandler$Recipe")
.