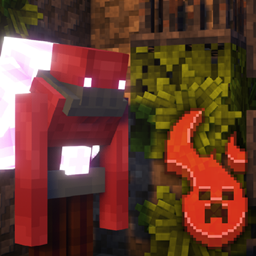
Some features I've implemented in kubejs
Rad586 opened this issue ยท 0 comments
I'm putting the script here so if someone wants it, they can use it now.
1. Random hit stun
In deadcells, the stun rate raries from weapon to weapon. It's too costy to implement the full feature, so here it goes randomly.
EntityEvents.hurt(event => {
//Only player
if (event.source.actual == null) return
if (!event.source.actual.isPlayer()) return
//Dont stun the boss
if (event.entity.type == "minecells:conjunctivius") return
//Only in minecells dimension
if (event.entity.level.dimension == 'minecells:insufferable_crypt' ||
event.entity.level.dimension == 'minecells:prison' ||
event.entity.level.dimension == 'minecells:promenade'){
//1/3 chance to stun for 16 ticks
if (event.getLevel().getRandom().nextInt(3) == 0){
event.entity.potionEffects.add('minecells:stunned', 16, 0, false, false)
}
}
})
2. Killing grants speed
In deadcells, killing grants speed. In minecraft, maybe we also need haste and resistance, since the speed effect makes player eaiser to get attacked.
EntityEvents.death(event => {
//Only player
if (event.source.actual == null) return
if (!event.source.actual.isPlayer()) return
//Only in minecells dimension
if (event.entity.level.dimension == 'minecells:insufferable_crypt' ||
event.entity.level.dimension == 'minecells:prison' ||
event.entity.level.dimension == 'minecells:promenade'){
++event.source.actual.persistentData.minecells
}
//3 kills = 23s speed effect
if (event.source.actual.persistentData.minecells >= 3){
event.source.actual.persistentData.minecells = 0
event.source.actual.potionEffects.add('minecraft:speed', 460, 0, false, false)
event.source.actual.potionEffects.add('minecraft:haste', 60, 1, false, false)
event.source.actual.potionEffects.add('minecraft:resistance', 460, 0, false, false)
}
})
3. Auto switch gamemode & attribute modifying
Build a two block column makes player safe, which is op, and adventure mode bans that.
Deadcells is fast paced, then in minecraft, it would be cool to attack and move faster.
Also, since the base attack of player is tweaked to -2, they can't punch mobs and stun them.
PlayerEvents.tick(event => {
//Dont check that often
if(event.player.age % 70 != 0) return
//Dont check if it's in creative mode
if(event.player.isCreative()) return
//Modify attributes and change gamemode in minecells dimensions
if (event.player.level.dimension == 'minecells:insufferable_crypt' ||
event.player.level.dimension == 'minecells:prison' ||
event.player.level.dimension == 'minecells:promenade'){
event.server.runCommandSilent(`gamemode adventure ${event.player.username}`)
//Move faster
event.server.runCommandSilent(`attribute ${event.player.username} minecraft:generic.movement_speed base set 0.1418`)
//Attack faster, but deals less damage
//No punching mobs
event.server.runCommandSilent(`attribute ${event.player.username} minecraft:generic.attack_speed base set 4.4`)
event.server.runCommandSilent(`attribute ${event.player.username} minecraft:generic.attack_damage base set -2`)
} else{
//Back to normal
event.server.runCommandSilent(`gamemode survival ${event.player.username}`)
event.server.runCommandSilent(`attribute ${event.player.username} minecraft:generic.movement_speed base set 0.1`)
event.server.runCommandSilent(`attribute ${event.player.username} minecraft:generic.attack_speed base set 4`)
event.server.runCommandSilent(`attribute ${event.player.username} minecraft:generic.attack_damage base set 1`)
}
})