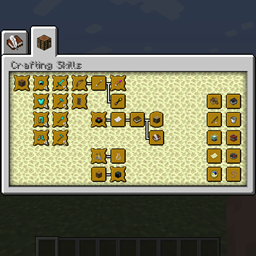
Free child skills
NightmareTwilight opened this issue ยท 0 comments
After buying the first parent skill in a group of skills all subsequent skills in the group becomes free. Posting code here since I'm using your template:
public class AttackSpeed extends AttackSpeedSkill {
public static final String[] NAME = new String[] { "atkSpeedI", "atkSpeedII", "atkSpeedIII", "atkSpeedIV" };
public static final Item[] ICON = new Item[] { Items.WOODEN_SWORD, Items.STONE_SWORD, Items.IRON_SWORD, Items.DIAMOND_SWORD};
public static final SkillAttributeModifier[] MODIFIERS = new SkillAttributeModifier[4];
protected static int TIER;
protected final int tier;
public AttackSpeed() {
super(NAME[(TIER = TIER >= NAME.length ? 0 : TIER)], ICON[TIER]);
this.tier = TIER;
this.addRequirement(new SkillPointRequirement(tier));
MODIFIERS[TIER] = new SkillAttributeModifier("attackSkill." + NAME[TIER], 1, 0);
TIER++;
}
@Override
public SkillAttributeModifier getModifier(EntityLivingBase entity, SkillBase skill) {
if (skill instanceof AttackSpeed)
return MODIFIERS[((AttackSpeed) skill).getTier()];
return null;
}
public int getTier() {
return this.tier;
}
@Override
public List<ISkillRequirment> getRequirments(EntityLivingBase entity, boolean hasSkill) {
return Collections.singletonList(new LevelRequirement(3+tier*3));
}
}
public class AttackSkillPage extends SkillPageBase {
private DoubleDamage doubleDamage;
private ExtraDamage extraDamage;
private ExtraDamage extraDamage1;
private ExtraDamage extraDamage2;
private ExtraDamage extraDamage3;
private ExtraDamage extraDamage4;
private ExtraDamage extraDamage5;
private AttackSpeed attackSpeed;
private AttackSpeed attackSpeed1;
private AttackSpeed attackSpeed2;
private AttackSpeed attackSpeed3;
private SwordProficiency swordProficiency;
private AxeProficiency axeProficiency;
public AttackSkillPage() {
super("AttackPage");
}
@Override
public void registerSkills() {
extraDamage = new ExtraDamage();
extraDamage1 = (ExtraDamage) new ExtraDamage().setParent(extraDamage);
extraDamage2 = (ExtraDamage) new ExtraDamage().setParent(extraDamage1);
extraDamage3 = (ExtraDamage) new ExtraDamage().setParent(extraDamage2);
extraDamage4 = (ExtraDamage) new ExtraDamage().setParent(extraDamage3);
extraDamage5 = (ExtraDamage) new ExtraDamage().setParent(extraDamage4);
doubleDamage = (DoubleDamage) new DoubleDamage().setParent(extraDamage4);
attackSpeed = new AttackSpeed();
attackSpeed1 = (AttackSpeed) new AttackSpeed().setParent(attackSpeed);
attackSpeed2 = (AttackSpeed) new AttackSpeed().setParent(attackSpeed1);
attackSpeed3 = (AttackSpeed) new AttackSpeed().setParent(attackSpeed2);
swordProficiency = new SwordProficiency();
axeProficiency = new AxeProficiency();
}
@Override
public void loadPage() {
addSkill(extraDamage, 0, 0);
addSkill(extraDamage1, 1, 0);
addSkill(extraDamage2, 2, 0);
addSkill(extraDamage3, 3, 0);
addSkill(extraDamage4, 4, 0);
addSkill(extraDamage5, 5, 0);
addSkill(doubleDamage, 5, 1);
addSkill(attackSpeed, 0, 2);
addSkill(attackSpeed1, 1, 2);
addSkill(attackSpeed2, 2, 2);
addSkill(attackSpeed3, 3, 2);
addSkill(swordProficiency, 0, 5);
addSkill(axeProficiency, 1, 5);
}
@Override
public BackgroundType getBackgroundType() {
return BackgroundType.NETHERRACK;
}
}