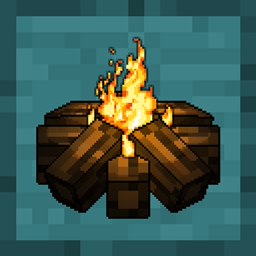
Biome Control
lynaevm opened this issue ยท 3 comments
It would be nice to have crafttweaker support for the effects that biome has on the drying time of items on the drying racks, as well as more visibility of what the default values for various biomes are.
I've inserted a snippet of the method that governs the drying rack speed. I've added comments to explain the logic at each step.
I can expose all of the hard-coded values visible in the snippet below pretty easily to the config files. Is there a reason that you are specifically asking for CrT support to change these values? Or would config values be acceptable?
Also, do you have any suggestions to increase the visibility of these values? Are you talking about in-game visibility or config-level visibility?
private float updateSpeed() {
float newSpeed;
Biome biome = this.world.getBiome(this.pos);
boolean canRain = biome.canRain();
if (canRain && this.world.isRainingAt(this.pos.up())) {
// If the biome can rain and the device is being directly rained on,
// set the new speed to this device's rain speed.
// The crude drying rack has a rain speed of 0 because the item is covered.
// The drying rack has a rain speed of -1 because the item is not covered.
// A negative speed will reduce recipe progress.
newSpeed = this.getRainSpeed();
} else if ((canRain && this.world.isRaining()) || biome.isHighHumidity()) {
// If the biome can rain and it is raining somewhere, or the biome is high
// humidity, the new speed is set to this:
newSpeed = 0.25f;
} else if (this.world.provider.isNether()) {
// If the device is in the Nether, set the new speed to this:
newSpeed = 2.0f;
} else {
// Otherwise, set the new speed to this:
newSpeed = 1.0f;
// And then additively modify it based on further criteria.
if (BiomeDictionary.getBiomes(BiomeDictionary.Type.HOT).contains(biome)) {
// If the biome is hot, increase the speed:
newSpeed += 0.2f;
}
if (BiomeDictionary.getBiomes(BiomeDictionary.Type.DRY).contains(biome)) {
// If the biome is dry, increase the speed:
newSpeed += 0.2f;
}
if (BiomeDictionary.getBiomes(BiomeDictionary.Type.COLD).contains(biome)) {
// If the biome is cold, decrease the speed:
newSpeed -= 0.2f;
}
if (BiomeDictionary.getBiomes(BiomeDictionary.Type.WET).contains(biome)) {
// If the biome is wet, decrease the speed:
newSpeed -= 0.2f;
}
}
// Next, scan a 2x2x2 region around the device and look for a fire block,
// or a block that counts as a fire source.
final float[] fireSourceBonus = new float[1];
BlockHelper.forBlocksInCube(this.world, this.pos, 2, 2, 2, (w, p, bs) -> {
if (this.isSpeedBonusBlock(bs, p)) {
// Add speed bonus for each adjacent fire or fire source block:
fireSourceBonus[0] += 0.2f;
}
return true;
});
newSpeed += fireSourceBonus[0];
if (!this.world.isRaining()
&& this.world.canSeeSky(this.pos.up())
&& this.world.getWorldTime() % 24000 > 3000
&& this.world.getWorldTime() % 24000 < 9000) {
// Add extra speed bonus if the rack it's not raining, the rack is
// under the sky, and it's daytime.
newSpeed += 0.2f;
}
// Finally, apply the base modifier from the config and return the
// new, calculated speed.
return this.getSpeedModified(newSpeed);
}
Thanks for this very complete information.
I asked for it for two reasons: other mods I'm using have some similar biome specs you can set and I was going to attempt consistency (tho they lack such a thorough way of setting their value) and because there are often mods that add a lot of biomes and not all of them have complete info, I think.
What you shared was exactly what I wanted for visibility -- for makers, not players. Config support vs crt support is all equal to me, I was just looking for a way to tweak things when the default values didn't seem to suit the situation, through no fault of pyrotech
For example: I am seeing a significant speed increase because of being in the nether. But I am using NetherEx and one of the biomes it adds is a frozen wasteland "arctic abyss" and I get the same speed boost there that I do in the most desert-like of the nether biomes. So even tho the algorithm is super smart, I would normally tweak that biome to be less efficient. I have also created custom defined biomes and not known how to set the heat/humidty/etc (clearly should learn).
This is also a low priority request. thanks for all your works and all the updates.
It sounds like a three-part solution is in order:
- expose the hard-coded speed values to configs, and
- implement CrT methods for overriding the base speed in specific biomes, and
- document the logic for makers, using clear identifiers that map to the config values
I imagine the CrT looking something like this:
import mods.pyrotech.DryingRack;
DryingRack.setBiomeSpeed(3.0, "minecraft:plains");
DryingRack.setBiomeSpeed(4.2, ["minecraft:ocean", "minecraft:desert"]);
Then the speed calculation logic would be altered to first look at the values specified by CrT before defaulting to the existing logic. The modifiers would still apply, like the fire source bonus and the daylight / not raining / can see sky bonus, but the CrT supplied values would be queried for a base value first.