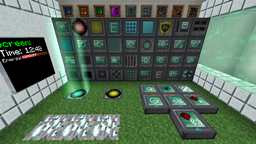
Support for more wrenches
planetguy32 opened this issue ยท 6 comments
It would be nice if we could use wrenches from various mods to adjust RFTools blocks - TE3 crescent hammers? IC2 wrenches? Railcraft crowbars? EnderIO yeta wrenches? In my own mod, I did it with reflection to avoid hard dependencies and make it fail gracefully. Here it is, feel free to borrow this code:
import java.util.ArrayList;
import java.util.List;
import net.minecraft.item.ItemStack;
public class WrenchChecker {
private static List<Class> wrenchClasses=new ArrayList<Class>();
//should call from Init or PostInit - all wrench classes should be loaded by then
public static void init(){
for(String className:new String[]{
/*
* Can add or remove class names here
* Use API interface names where possible, in case of refactoring
* note that many mods implement BC wrench API iff BC is installed
* and include no wrench API of their own - we use implementation
* classes here to catch these cases.
*/
"buildcraft.api.tools.IToolWrench", //Buildcraft
"resonant.core.content.ItemScrewdriver", //Resonant Induction
"ic2.core.item.tool.ItemToolWrench", //IC2
"ic2.core.item.tool.ItemToolWrenchElectric", //IC2 (more)
"mrtjp.projectred.api.IScrewdriver", //Project Red
"mods.railcraft.api.core.items.IToolCrowbar", //Railcraft
"com.bluepowermod.items.ItemScrewdriver", //BluePower
"cofh.api.item.IToolHammer", //Thermal Expansion and compatible
"appeng.items.tools.quartz.ToolQuartzWrench", //Applied Energistics
"crazypants.enderio.api.tool.ITool", //Ender IO
"mekanism.api.IMekWrench", //Mekanism
}){
try {
wrenchClasses.add(Class.forName(className));
} catch (ClassNotFoundException e) {
Debug.dbg("Failed to load wrench class "+className);
}
}
}
public static boolean isAWrench(ItemStack stk){
for(Class c:wrenchClasses){ //must iterate - testing isAssignableFrom, not equals
if(stk !=null && stk.getItem() !=null && stk.getItem().getClass().isAssignableFrom(c))
return true;
}
return false;
}
}
I found a Load Order Bug on 1.7.10, not sure if it's been fixed in later version, but I only play on 1.7.10, and even though you don't support 1.7.10, I figure I should at least create a proper Bug Tracker for it should anyone decide to apply a fix somehow. I have submitted it as Issue #1180
Don't implement all those wrenches either the COFH or buildcraft wrench interface because I do support those two. I thought that would be sufficient to support all those.
Many of the mods which implement the BC wrench API have an Optional annotation that removes it if Buildcraft's not installed, and this approach makes them work without Buildcraft by specifying the wrench item's class. I haven't seen any mods implementing the CoFH wrench API (maybe it's a Thermal Expansion thing), but I'd assume the situation isn't much better. Some of these (IIRC BluePower and Project:Red screwdrivers) don't implement any special API at all, so an approach like this is required for them. Adding support for a new wrench type doesn't require building against the API or introduce a hard dependency - it could even be made available as a config property for people with lesser-known mods.
A couple more wrenches I've found:
pneumaticCraft.common.item.ItemPneumaticWrench
powercrystals.minefactoryreloaded.api.IToolHammer
You can probably remove the Calclavia wrench because the class has been repackaged since I added the entry, and Resonant Core has no Optional on the Buildcraft wrench API so it will always be covered by the BC check.