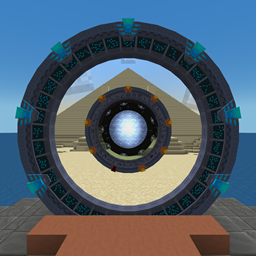
Manual dialing with computer
DragingDeezNutts opened this issue · 1 comments
How do I give computer the commands for SG? It’s my first time working with CC
Well, firstly, if you can, I suggest you look at some CC-Tweaked tutorials just get some basic understanding of how it works
If you generally dislike coding, no worries, I'll be making a Dialing Computer like the one in SGC eventually, though it will take a while.
Anyway, let's get to it. The code I'll be showing is from a very primitive example of a ComputerCraft program I made a while ago, written in like 10 minutes, so don't expect too much.
https://github.com/Povstalec/StargateJourney-ComputerCraft-Programs/blob/main/StargateDialing.lua
(I'll be using -- to make comments on the code written above the --)
interface = peripheral.wrap("basic_interface_2")
-- This connects the interface to the computer. You're not actually connecting the computer to the Stargate, but forwarding the commands through the Basic Interface.
-- The number for you might be different, but you can check it simply by right clicking the modem you use to connect the Basic Interface.
function dial(address)
local addressLength = #address
local start = interface.getChevronsEngaged() + 1
for chevron = start,addressLength,1
do
-- This whole loop has 3 functions:
-- 1. Rotate
local symbol = address[chevron]
if chevron % 2 == 0 then
-- This decides if the Stargate should rotate clockwise or counter-clockwise.
-- Clockwise if the chevron number is even, counter-clockwise when it's odd
interface.rotateClockwise(symbol)
else
interface.rotateAntiClockwise(symbol)
end
while(not interface.isCurrentSymbol(symbol))
do
print("Feedback",interface.getRecentFeedback())
sleep(0)
end
-- This whole while loop is pretty much pointless and just for show. It only really monitors the state of the gate, but doesn't actually control anything
sleep(1)
print("Code",interface.raiseChevron())
-- This raises the chevron and gives you back its status
sleep(1)
print("Code",interface.lowerChevron())
-- This lowers the chevron (which encodes the symbol) and gives you back its status
sleep(1)
end
end
abydosAddress = {26,6,14,31,11,29,0}
chulakAddress = {8,1,22,14,36,19,0}
lanteaAddress = {18,20,1,15,14,7,19,0}
-- These are the addresses, you can add more by just expanding this section
print("Avaiting input:")
print("1 = Abydos")
print("2 = Chulak")
print("3 = Lantea")
input = tonumber(io.read())
if input == 1 then
dial(abydosAddress)
elseif input == 2 then
dial(chulakAddress)
elseif input == 3 then
dial(lanteaAddress)
end
-- This whole if-elseif segment just serves to let you decide which address to dial. You can add more options by just expanding on what's already written there
There are more commands I have not mentioned, which you can read here:
https://github.com/Povstalec/StargateJourney/wiki/Interfaces#interface-commands