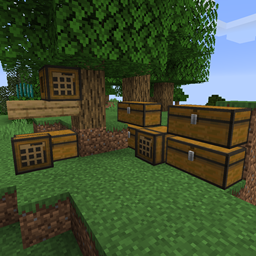
Enhancement: Take out from last discovered to first discovered inventory
dmartin-tech opened this issue ยท 1 comments
What is expected: Taking items out of the system will take from the chests discovered latest to the chests that were discovered first.
What happens: Taking items out of the system it uses the same check that is used to place the item into the inventory in order to choose one to pull from, causing the chests further down the chain to have partial stacks that could be removed, but are not. There could be instances where partial stacks are put into the system, but are not combined and as a result will waste space.
Potential complications: performance, missing items/duplication of items.
The Forge code would be simple to change with reversing the loop. For the Fabric code, I think making a separate method to handle a reversed version would help keep the code clean.
Currently on Fabric I've experimented with (as a proof of concept):
private boolean calling;
public <R> R call(BiFunction<InventoryWrapper, Integer, R> func, int slot, R def, boolean reversed) {
if(calling)return def;
calling = true;
if(!reversed) {
for (int i = 0; i < invSizes.length; i++) {
if (slot >= invSizes[i]) slot -= invSizes[i];
else {
R r = func.apply(handlers.get(i), slot);
calling = false;
return r;
}
}
}
else {
for (int i = invSizes.length-1; i >= 0; i--) {
if (slot >= invSizes[i]) slot -= invSizes[i];
else {
R r = func.apply(handlers.get(i), slot);
calling = false;
return r;
}
}
}
calling = false;
return def;
}
The methods using the reversed versions (as far as I am aware) would be: getStack() and removeStack()
The next hurdle is trying to pull the last slot of the inventory with the item.