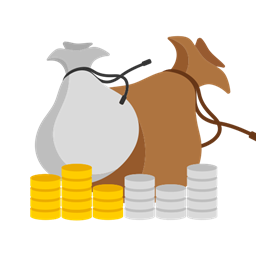
Request: tooltip prices for other realms if scanned
Jahfry opened this issue ยท 1 comments
What version(s) of WoW are you using?
Retail
What problem are you experiencing that led to you asking for this feature?
I play on a few realms. Warband Bank gives the ability to easily list items on other realms.
What solution would you like?
I'd love to be able to add multiple realms to show most recently scanned price for items. I wouldn't mind needing to type in the specific realm name if a drop-down is a pain to make.
Any alternatives you can think of?
If this already exists, I just didn't see the method, pointer would be welcome.
Anything else?
No response
Founded Solutions. But I cant support this code.Better have this code in Auctionator.
First simple addon code
local DB = "AuctionWhereDB"
local function computeTable(...)
local t, ks
if type(...) == "table" then
t, ks = ..., { select(2, ...) }
else
t, ks = _G, { ... }
end
for _, k in ipairs(ks) do
t[k] = t[k] or {}
t = t[k]
end
return t
end
local ITEM = "ITEM"
local CHARACTER = "CHARACTER"
local CONNECTED_REALM_TO_CHARACTER = "CONNECTED_REALM_TO_CHARACTER"
local function computeRealm()
local realms = GetAutoCompleteRealms()
table.sort(realms)
return realms[1] or GetNormalizedRealmName()
end
local function processBrowseResult(result)
computeTable(DB, ITEM, result.itemKey.itemID)[computeRealm()] = { price = result.minPrice, time = time() }
end
local function processCommoditySearchResult(result)
computeTable(DB, ITEM, result.itemID)[computeRealm()] = { price = result.unitPrice, time = time() }
end
local function processItemSearchResult(result)
computeTable(DB, ITEM, result.itemKey.itemID)[computeRealm()] = { price = result.buyoutAmount, time = time() }
end
local f = CreateFrame("Frame")
f:RegisterEvent("PLAYER_ENTERING_WORLD")
f:RegisterEvent("AUCTION_HOUSE_BROWSE_RESULTS_UPDATED")
f:RegisterEvent("AUCTION_HOUSE_BROWSE_RESULTS_ADDED")
f:RegisterEvent("COMMODITY_SEARCH_RESULTS_UPDATED")
f:RegisterEvent("ITEM_SEARCH_RESULTS_UPDATED")
f:SetScript("OnEvent", function(_, event, ...)
if event == "PLAYER_ENTERING_WORLD" then
f:UnregisterEvent("PLAYER_ENTERING_WORLD")
local realm = GetNormalizedRealmName()
local player = UnitName("player") .. "-" .. realm
local _, class = UnitClass("player")
computeTable(DB, CHARACTER)[player] = { realm = realm, class = class }
computeTable(DB, CONNECTED_REALM_TO_CHARACTER, realm)[player] = true
for _, connectedRealm in ipairs(GetAutoCompleteRealms()) do
computeTable(DB, CONNECTED_REALM_TO_CHARACTER, connectedRealm)[player] = true
end
local now = time()
local sevenDays = 3 * 24 * 60 * 60
for _, itemDB in pairs(computeTable(DB, ITEM)) do
for k, data in pairs(itemDB) do
if now - data.time > sevenDays then
itemDB[k] = nil
end
end
end
end
if event == "AUCTION_HOUSE_BROWSE_RESULTS_UPDATED" then
for _, result in ipairs(C_AuctionHouse.GetBrowseResults()) do
processBrowseResult(result)
end
end
if event == "AUCTION_HOUSE_BROWSE_RESULTS_ADDED" then
for _, result in ipairs(...) do
processBrowseResult(result)
end
end
if event == "COMMODITY_SEARCH_RESULTS_UPDATED" then
local result = C_AuctionHouse.GetCommoditySearchResultInfo(..., 1)
if result then
processCommoditySearchResult(result)
end
end
if event == "ITEM_SEARCH_RESULTS_UPDATED" then
local result = C_AuctionHouse.GetItemSearchResultInfo(..., 1)
if result then
processItemSearchResult(result)
end
end
end)
local function elapsed(t)
local dt = time() - t
if dt < 60 then return dt .. " seconds ago" end
dt = floor(dt / 60)
if dt < 60 then return dt .. " minutes ago" end
dt = floor(dt / 60)
if dt < 24 then return dt .. " hours ago" end
dt = floor(dt / 24)
return dt .. " days ago"
end
TooltipDataProcessor.AddTooltipPostCall(Enum.TooltipDataType.Item, function(tooltip, data)
local array = GetPairsArray(computeTable(DB, ITEM, data.id))
table.sort(array, function(a, b) return a.value.price < b.value.price end)
for _, d in ipairs(array) do
tooltip:AddLine(" ")
tooltip:AddDoubleLine(
d.key .. " " .. WHITE_FONT_COLOR:WrapTextInColorCode(elapsed(d.value.time)),
WHITE_FONT_COLOR:WrapTextInColorCode(GetMoneyString(d.value.price, true)))
for player in pairs(computeTable(DB, CONNECTED_REALM_TO_CHARACTER, d.key)) do
local character = computeTable(DB, CHARACTER, player)
tooltip:AddLine(RAID_CLASS_COLORS[character.class]:WrapTextInColorCode(player))
end
end
end)
Second Simple addon text Warband Sync only Favorites in Auction Main frame
local addonName = ...
local gdb, cdb
local function serializeValues(t)
local keys, values = {}, {}
for k in pairs(t) do table.insert(keys, k) end
table.sort(keys)
for _, k in ipairs(keys) do table.insert(values, t[k]) end
return table.concat(values, "-")
end
local function sync(itemKey)
local key = serializeValues(itemKey)
if not gdb.favorites[key] == not cdb.favorites[key] then
return false
end
C_AuctionHouse.SetFavoriteItem(itemKey, gdb.favorites[key] ~= nil)
return true
end
local function setFavorite(itemKey, favorite)
local key = serializeValues(itemKey)
gdb.favorites[key] = favorite and itemKey or nil
cdb.favorites[key] = favorite and itemKey or nil
end
hooksecurefunc(C_AuctionHouse, "SetFavoriteItem", setFavorite)
local f = CreateFrame("Frame")
f:RegisterEvent("ADDON_LOADED")
f:RegisterEvent("AUCTION_HOUSE_SHOW")
f:RegisterEvent("AUCTION_HOUSE_CLOSED")
f:SetScript("OnEvent", function(_, event, ...)
if event == "ADDON_LOADED" and ... == addonName then
f:UnregisterEvent("ADDON_LOADED")
AuctionFavoritesSyncGDB = AuctionFavoritesSyncGDB or {}
gdb = AuctionFavoritesSyncGDB
gdb.favorites = gdb.favorites or {}
AuctionFavoritesSyncCDB = AuctionFavoritesSyncCDB or {}
cdb = AuctionFavoritesSyncCDB
cdb.favorites = cdb.favorites or {}
if not cdb.sync then
f:RegisterEvent("AUCTION_HOUSE_BROWSE_RESULTS_UPDATED")
f:RegisterEvent("AUCTION_HOUSE_BROWSE_RESULTS_ADDED")
f:RegisterEvent("COMMODITY_SEARCH_RESULTS_UPDATED")
f:RegisterEvent("COMMODITY_SEARCH_RESULTS_ADDED")
f:RegisterEvent("ITEM_SEARCH_RESULTS_UPDATED")
f:RegisterEvent("ITEM_SEARCH_RESULTS_ADDED")
end
end
if event == "AUCTION_HOUSE_SHOW" then
local needRefresh = false
if cdb.sync then
for _, favorites in ipairs { gdb.favorites, cdb.favorites } do
for _, itemKey in pairs(favorites) do
needRefresh = sync(itemKey) or needRefresh
end
end
else
for _, itemKey in pairs(gdb.favorites) do
C_AuctionHouse.SetFavoriteItem(itemKey, true)
needRefresh = true
end
end
if needRefresh then
C_AuctionHouse.SearchForFavorites({})
end
end
if event == "AUCTION_HOUSE_CLOSED" then
cdb.sync = true
f:UnregisterAllEvents()
end
local function processItemKey(itemKey)
setFavorite(itemKey, C_AuctionHouse.IsFavoriteItem(itemKey))
end
if event == "AUCTION_HOUSE_BROWSE_RESULTS_UPDATED" then
for _, result in ipairs(C_AuctionHouse.GetBrowseResults()) do
processItemKey(result.itemKey)
end
end
if event == "AUCTION_HOUSE_BROWSE_RESULTS_ADDED" then
for _, result in ipairs(...) do
processItemKey(result.itemKey)
end
end
if event == "COMMODITY_SEARCH_RESULTS_UPDATED" or event == "COMMODITY_SEARCH_RESULTS_ADDED" then
processItemKey(C_AuctionHouse.MakeItemKey(...))
end
if event == "ITEM_SEARCH_RESULTS_UPDATED" or event == "ITEM_SEARCH_RESULTS_ADDED" then
processItemKey(...)
end
end)