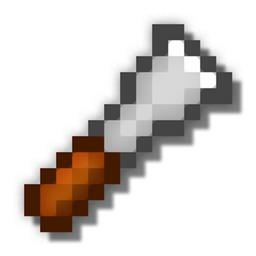
Can't create another chisel with API
tgodoib opened this issue ยท 1 comments
I've been trying to create an Electrical Chisel, but when i try to open the inventory by right clicking with the chisel i made, the window opens and closes immediately, left clicking to chisel a block works though. Is that an Api problem, or am i doing something wrong?
Main class and Item Class:
@Mod(modid = "echisel", version = "1.0")
public class ElectricChisel {
public static ItemElectricChisel echisel;
@Mod.EventHandler
public void preInit(FMLPreInitializationEvent e){
echisel = new ItemElectricChisel();
GameRegistry.register(echisel);
ModelLoader.setCustomModelResourceLocation(echisel, 0, new ModelResourceLocation("echisel:electric_chisel", "inventory"));
}
}
public ItemElectricChisel(){
super();
setMaxDamage(1000);
setCreativeTab(CreativeTabs.TOOLS);
setMaxStackSize(1);
setUnlocalizedName("item_electric_chisel");
setRegistryName("item_electric_chisel_registry");
}
@Override
public int getMaxDamage() {
return 1000;
}
@Override
public boolean isDamageable() {
return true;
}
@SuppressWarnings({ "unchecked", "rawtypes" })
@Override
public void addInformation(ItemStack stack, EntityPlayer player, List list, boolean held) {
String base = "item.chisel.chisel.desc.";
String gui = I18n.format(base + "gui");
list.add(gui);
}
@Override
public boolean isFull3D() {
return true;
}
@Override
public Multimap<String, AttributeModifier> getAttributeModifiers(EntityEquipmentSlot slot, ItemStack stack) {
Multimap<String, AttributeModifier> multimap = HashMultimap.create();
if (slot == EntityEquipmentSlot.MAINHAND) {
multimap.put(SharedMonsterAttributes.ATTACK_DAMAGE.getAttributeUnlocalizedName(), new AttributeModifier(ATTACK_DAMAGE_MODIFIER, "Chisel Damage", 2, 0));
}
return multimap;
}
@Override
public boolean canOpenGui(World world, EntityPlayer player, EnumHand hand) {
return true;
}
@Override
public IChiselGuiType getGuiType(World world, EntityPlayer player, EnumHand hand) {
return IChiselGuiType.ChiselGuiType.NORMAL;
}
@Override
public boolean onChisel(World world, EntityPlayer player, ItemStack chisel, ICarvingVariation target) {
return true;
}
@Override
public boolean canChisel(World world, EntityPlayer player, ItemStack chisel, ICarvingVariation target) {
return true;
}
@Override
public boolean canChiselBlock(World world, EntityPlayer player, EnumHand hand, BlockPos pos, IBlockState state) {
return true;
}
@Override
public boolean hasModes(EntityPlayer player, EnumHand hand) {
return false;
}
@Override
public boolean shouldCauseReequipAnimation(ItemStack oldStack, ItemStack newStack, boolean slotChanged) {
return slotChanged || !ItemStack.areItemsEqual(oldStack, newStack);
}