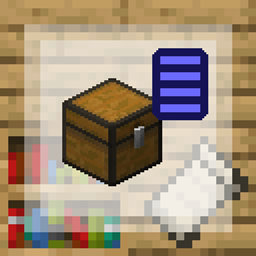
How to wrap Inventory or SidedInventory as ItemInsertable or ItemExtractable
Phoupraw opened this issue ยท 2 comments
I want to deal with those using vanilla API (implemented Inventory or SidedInventory) and those using LibBlockAttributes (implemented ItemInsertable or ItemExtractable) in a "LibBlockAttributes" way. There are some seemingly relative classes and interfaces, but they either are not implemented ItemInsertable or ItemExtractable (such as FixedItemInv, FixedInventoryVanillaWrapper, FixedSidedInventoryVanillaWrapper), or can't easily wrap Inventory or SidedInventory (such as DirectFixedItemInv).
Is there any way to easily wrap Inventory or SidedInventory as ItemInsertable or ItemExtractable?
Yes - the conversion chain is:
Inventory inv = // however you get this
FixedItemInv wrapper = new FixedInventoryVanillaWrapper(inv);
ItemInsertable insertable = wrapper.getInsertable();
ItemExtractable extractable = wrapper.getExtractable();
More generally, any FixedItemInv
can be converted into a GroupedItemInv
via FixedItemInv.getGrouped()
- or directly into ItemInsertable
or ItemExtractable
via getInsertable()
and getExtractable()
.
(The reason for this separation is simple: some blocks - like a vanilla chest - can be accessed either by individual slots (FixedItemInv), or you can ignore slots and just see the number of items in a chest (GroupedItemInv). However other blocks (like a barrel which holds a large number of a single stack) don't really make sense when accessed from a single slot. Some other blocks - like a buildcraft pipe - can't be accessed at all, and can only have items inserted into it.
If the inventory or sided inventory comes out of the world (for example it's a block) then you can use LBA's lookup methods to do the conversion automatically:
World world = // wherever you get the world from
BlockPos targetPos = // Whatever block you want to insert into
Direction direction = // The direction to search in - this is the opposite of the side of the block you'd get from
ItemInsertable insertable = ItemAttributes.INSERTABLE.get(world, targetPos, SearchOptions.inDirection(direction));
ItemExtractable extractable = ItemAttributes.EXTRACTABLE.get(world, targetPos, SearchOptions.inDirection(direction));
// If you're doing this from a `BlockEntity` then you can use `getFromNeighbour(BlockEntity, Direction) instead
Yes - the conversion chain is:
Inventory inv = // however you get this FixedItemInv wrapper = new FixedInventoryVanillaWrapper(inv); ItemInsertable insertable = wrapper.getInsertable(); ItemExtractable extractable = wrapper.getExtractable();More generally, any
FixedItemInv
can be converted into aGroupedItemInv
viaFixedItemInv.getGrouped()
- or directly intoItemInsertable
orItemExtractable
viagetInsertable()
andgetExtractable()
.(The reason for this separation is simple: some blocks - like a vanilla chest - can be accessed either by individual slots (FixedItemInv), or you can ignore slots and just see the number of items in a chest (GroupedItemInv). However other blocks (like a barrel which holds a large number of a single stack) don't really make sense when accessed from a single slot. Some other blocks - like a buildcraft pipe - can't be accessed at all, and can only have items inserted into it.
If the inventory or sided inventory comes out of the world (for example it's a block) then you can use LBA's lookup methods to do the conversion automatically:
World world = // wherever you get the world from BlockPos targetPos = // Whatever block you want to insert into Direction direction = // The direction to search in - this is the opposite of the side of the block you'd get from ItemInsertable insertable = ItemAttributes.INSERTABLE.get(world, targetPos, SearchOptions.inDirection(direction)); ItemExtractable extractable = ItemAttributes.EXTRACTABLE.get(world, targetPos, SearchOptions.inDirection(direction)); // If you're doing this from a `BlockEntity` then you can use `getFromNeighbour(BlockEntity, Direction) instead
Wow, there are lots of methods regard of vanilla API that I haven't discovered. Thank you for telling me these.