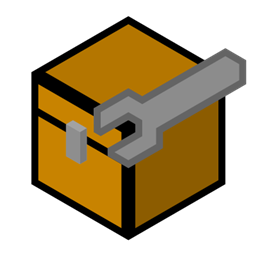
[Bug] Crash on world load - Attempt to modify after being frozen
Opened this issue · 9 comments
Description: Error log states that the instance crashed, MC stuck on loading terrain screen.
Steps to reproduce: Add the script and create/load a world.
Script Used:
import loottweaker.vanilla.loot.LootTables;
import loottweaker.vanilla.loot.LootTable;
import loottweaker.vanilla.loot.LootPool;
import loottweaker.vanilla.loot.Conditions;
import loottweaker.vanilla.loot.Functions;
# ==================================================
# Definitions
# ==================================================
val blaze = LootTables.getTable("minecraft:entities/blaze");
val cave_spider = LootTables.getTable("minecraft:entities/cave_spider");
val creeper = LootTables.getTable("minecraft:entities/creeper");
val enderman = LootTables.getTable("minecraft:entities/enderman");
val ghast = LootTables.getTable("minecraft:entities/ghast");
val guardian = LootTables.getTable("minecraft:entities/guardian");
val husk = LootTables.getTable("minecraft:entities/husk");
val iron_golem = LootTables.getTable("minecraft:entities/iron_golem");
val magma_cube = LootTables.getTable("minecraft:entities/magma_cube");
val shulker = LootTables.getTable("minecraft:entities/shulker");
val silverfish = LootTables.getTable("minecraft:entities/silverfish");
val skeleton = LootTables.getTable("minecraft:entities/skeleton");
val slime = LootTables.getTable("minecraft:entities/slime");
val spider = LootTables.getTable("minecraft:entities/spider");
val stray = LootTables.getTable("minecraft:entities/stray");
val witch = LootTables.getTable("minecraft:entities/witch");
val wither_skeleton = LootTables.getTable("minecraft:entities/wither_skeleton");
val zombie = LootTables.getTable("minecraft:entities/zombie");
val zombie_pigman = LootTables.getTable("minecraft:entities/zombie_pigman");
val zombie_villager = LootTables.getTable("minecraft:entities/zombie_villager");
# ==================================================
# Removal of Mined Resources
# ==================================================
// Husk
val p1Husk = husk.getPool("pool1");
p1Husk.removeItemEntry(<minecraft:iron_ingot>);
// Iron Golem
iron_golem.removePool("pool1");
// Witch
val pWitch = witch.getPool("main");
pWitch.removeItemEntry(<minecraft:redstone>);
pWitch.removeItemEntry(<minecraft:glowstone_dust>);
// Wither Skeleton
val pWSkeleton = wither_skeleton.getPool("main");
pWSkeleton.removeItemEntry(<minecraft:coal:0>);
// Zombie Pigman
zombie_pigman.removePool("pool1");
zombie_pigman.removePool("pool2");
// Zombie Villager
val p1VZombie = zombie_villager.getPool("pool1");
p1VZombie.removeItemEntry(<minecraft:iron_ingot>);
# ==================================================
# Bottle o' Enchanting Addition
# ==================================================
// Blaze
val pe1Blaze = blaze.addPool("extra1", 1, 1, 0, 1);
pe1Blaze.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Cave Spider
val pe1CSpider = cave_spider.addPool("extra1", 1, 1, 0, 1);
pe1CSpider.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Creeper
val pe1Creeper = creeper.addPool("extra1", 1, 1, 0, 1);
pe1Creeper.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Enderman
val pe1Enderman = enderman.addPool("extra1", 1, 1, 0, 1);
pe1Enderman.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Ghast
val pe1Ghast = ghast.addPool("extra1", 1, 1, 0, 1);
pe1Ghast.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Guardian
val pe1Guardian = guardian.addPool("extra1", 1, 1, 0, 1);
pe1Guardian.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Husk
val pe1Husk = husk.addPool("extra1", 1, 1, 0, 1);
pe1Husk.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Magma Cube
val pe1MCube = magma_cube.addPool("extra1", 1, 1, 0, 1);
pe1MCube.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Silverfish
val pe1Silverfish = silverfish.addPool("extra1", 1, 1, 0, 1);
pe1Silverfish.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Skeleton
val pe1Skeleton = skeleton.addPool("extra1", 1, 1, 0, 1);
pe1Skeleton.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Slime
val pe1Slime = slime.addPool("extra1", 1, 1, 0, 1);
pe1Slime.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Spider
val pe1Spider = spider.addPool("extra1", 1, 1, 0, 1);
pe1Spider.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Stray
val pe1Stray = stray.addPool("extra1", 1, 1, 0, 1);
pe1Stray.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Witch
val pe1Witch = witch.addPool("extra1", 1, 1, 0, 1);
pe1Witch.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Wither Skeleton
val pe1WSkeleton = wither_skeleton.addPool("extra1", 1, 1, 0, 1);
pe1WSkeleton.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Zombie
val pe1Zombie = zombie.addPool("extra1", 1, 1, 0, 1);
pe1Zombie.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Zombie Pigman
val pe1PZombie = zombie_pigman.addPool("extra1", 1, 1, 0, 1);
pe1PZombie.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
// Zombie Villager
val pe1VZombie = zombie_villager.addPool("extra1", 1, 1, 0, 1);
pe1VZombie.addItemEntry(<minecraft:experience_bottle>*10, 1, 0);
# ==================================================
# Mob Loot Buff
# ==================================================
// Blaze
val pe2Blaze = blaze.addPool("extra2", 1, 2, 1, 2);
pe2Blaze.addItemEntry(<minecraft:blaze_rod>*8, 1, 1);
// Cave Spider
val pe2CSpider = cave_spider.addPool("extra2", 1, 2, 1, 2);
pe2CSpider.addItemEntry(<minecraft:string>*16, 2, 1);
pe2CSpider.addItemEntry(<minecraft:spider_eye>*3, 1, 1);
// Creeper
val pe2Creeper = creeper.addPool("extra2", 1, 2, 1, 2);
pe2Creeper.addItemEntry(<minecraft:gunpowder>*16, 1, 1);
// Enderman
val pe2Enderman = enderman.addPool("extra2", 1, 2, 1, 2);
pe2Enderman.addItemEntry(<minecraft:ender_pearl>*8, 1, 1);
// Ghast
val pe2Ghast = ghast.addPool("extra2", 1, 2, 1, 2);
pe2Ghast.addItemEntry(<minecraft:ghast_tear>*2, 1, 1);
pe2Ghast.addItemEntry(<minecraft:gunpowder>*16, 2, 1);
// Guardian
val pe2Guardian = guardian.addPool("extra2", 1, 2, 1, 2);
pe2Guardian.addItemEntry(<minecraft:prismarine_shard>*16, 4, 1);
pe2Guardian.addItemEntry(<minecraft:prismarine_crystals>*2, 2, 1);
pe2Guardian.addItemEntry(<minecraft:sponge>*2, 1, 1);
// Husk
val pe2Husk = husk.addPool("extra2", 1, 2, 1, 2);
pe2Husk.addItemEntry(<minecraft:rotten_flesh>*16, 1, 1);
// Magma Cube
val pe2MCube = magma_cube.addPool("extra2", 1, 2, 1, 2);
pe2MCube.addItemEntry(<minecraft:magma_cream>*2, 1, 1);
// Shulker
val pe2Shulker = shulker.addPool("extra2", 1, 2, 1, 2);
pe2Shulker.addItemEntry(<minecraft:shulker_shell>*16, 1, 1);
// Skeleton
val pe2Skeleton = skeleton.addPool("extra2", 1, 2, 1, 2);
pe2Skeleton.addItemEntry(<minecraft:bone>*16, 1, 1);
pe2Skeleton.addItemEntry(<minecraft:arrow>*16, 1, 1);
// Slime
val pe2Slime = slime.addPool("extra2", 1, 2, 1, 2);
pe2Slime.addItemEntry(<minecraft:slime_ball>*16, 1, 1);
// Spider
val pe2Spider = spider.addPool("extra2", 1, 2, 1, 2);
pe2Spider.addItemEntry(<minecraft:string>*16, 2, 1);
pe2Spider.addItemEntry(<minecraft:spider_eye>*3, 1, 1);
// Stray
val pe2Stray = stray.addPool("extra2", 1, 2, 1, 2);
pe2Stray.addItemEntry(<minecraft:bone>*16, 1, 1);
// Witch
val pe2Witch = witch.addPool("extra2", 1, 3, 1, 2);
pe2Witch.addItemEntry(<minecraft:glass_bottle>*2, 1, 1, [], [Conditions.killedByPlayer()]);
pe2Witch.addItemEntry(<minecraft:gunpowder>*2, 1, 1, [], [Conditions.killedByPlayer()]);
pe2Witch.addItemEntry(<minecraft:spider_eye>*2, 1, 1, [], [Conditions.killedByPlayer()]);
pe2Witch.addItemEntry(<minecraft:sugar>*2, 1, 1, [], [Conditions.killedByPlayer()]);
pe2Witch.addItemEntry(<minecraft:stick>*2, 2, 1, [], [Conditions.killedByPlayer()]);
// Wither Skeleton
val pe2WSkeleton = wither_skeleton.addPool("extra2", 0, 1, 0, 1);
pe2WSkeleton.addItemEntry(<minecraft:bone>*16, 20, 1, [], [Conditions.killedByPlayer()]);
pe2WSkeleton.addItemEntry(<minecraft:skull:1>*1, 10, 1, [], [Conditions.killedByPlayer()]);
// Zombie
val pe2Zombie = zombie.addPool("extra2", 1, 2, 1, 2);
pe2Zombie.addItemEntry(<minecraft:rotten_flesh>*16, 1, 1);
// Zombie Pigman
val pe2PZombie = zombie_pigman.addPool("extra2", 1, 2, 1, 2);
pe2PZombie.addItemEntry(<minecraft:rotten_flesh>*16, 1, 1);
// Zombie Villager
val pe2VZombie = zombie_villager.addPool("extra2", 1, 2, 1, 2);
pe2VZombie.addItemEntry(<minecraft:rotten_flesh>*16, 1, 1);
LootTweaker: 1.12-0.0.6.3
CraftTweaker: 1.12-4.0.3
Forge: 1.12-14.21.1.2443
Minecraft: 1.12
Error Log:
---- Minecraft Crash Report ----
WARNING: coremods are present:
PLCore (PassableLeaves-1.12-6.1.0.0.jar)
LoadingPlugin (Quark-r1.2-104.jar)
AstralCore (astralsorcery-1.12-1.6.1.jar)
TNTUtilities Core (tnt_utilities-mc1.12-1.2.2.jar)
MalisisCorePlugin (malisiscore-1.12-6.0.2.jar)
MC64836CoreMod (mc64836-0.1.1.jar)
DummyPlugin (ForgeEndertech-1.12-3.0.0.0-build.0002.jar)
LoadingPlugin (NoNausea-MC1.12-1.0.jar)
DynamicSurroundingsCore (DynamicSurroundings-1.12-3.4.6.3.jar)
Contact their authors BEFORE contacting forge
// This is a token for 1 free hug. Redeem at your nearest Mojangsta: [~~HUG~~]
Time: 8/19/17 10:33 AM
Description: Exception in server tick loop
com.google.common.util.concurrent.UncheckedExecutionException: java.lang.RuntimeException: Attempted to modify LootPool after being frozen!
at com.google.common.cache.LocalCache$Segment.get(LocalCache.java:2217)
at com.google.common.cache.LocalCache.get(LocalCache.java:4154)
at com.google.common.cache.LocalCache.getOrLoad(LocalCache.java:4158)
at com.google.common.cache.LocalCache$LocalLoadingCache.get(LocalCache.java:5147)
at com.google.common.cache.LocalCache$LocalLoadingCache.getUnchecked(LocalCache.java:5153)
at net.minecraft.world.storage.loot.LootTableManager.func_186521_a(LootTableManager.java:40)
at net.minecraft.world.storage.loot.LootTableManager.func_186522_a(LootTableManager.java:49)
at net.minecraft.world.storage.loot.LootTableManager.<init>(LootTableManager.java:35)
at net.minecraft.world.WorldServer.func_175643_b(WorldServer.java:154)
at net.minecraft.server.integrated.IntegratedServer.func_71247_a(IntegratedServer.java:82)
at net.minecraft.server.integrated.IntegratedServer.func_71197_b(IntegratedServer.java:196)
at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:483)
at java.lang.Thread.run(Unknown Source)
Caused by: java.lang.RuntimeException: Attempted to modify LootPool after being frozen!
at net.minecraft.world.storage.loot.LootPool.checkFrozen(LootPool.java:98)
at net.minecraft.world.storage.loot.LootPool.addEntry(LootPool.java:129)
at leviathan143.loottweaker.common.zenscript.ZenLootPoolWrapper$AddLootEntry.applyTweak(ZenLootPoolWrapper.java:226)
at leviathan143.loottweaker.common.zenscript.ZenLootPoolWrapper$AddLootEntry.applyTweak(ZenLootPoolWrapper.java:202)
at leviathan143.loottweaker.common.zenscript.ZenLootPoolWrapper.applyLootTweaks(ZenLootPoolWrapper.java:193)
at leviathan143.loottweaker.common.zenscript.ZenLootTableWrapper$AddPool.applyTweak(ZenLootTableWrapper.java:126)
at leviathan143.loottweaker.common.zenscript.ZenLootTableWrapper$AddPool.applyTweak(ZenLootTableWrapper.java:103)
at leviathan143.loottweaker.common.zenscript.ZenLootTableWrapper.applyLootTweaks(ZenLootTableWrapper.java:83)
at leviathan143.loottweaker.common.tweakers.loot.LootTableTweaker.applyTweaks(LootTableTweaker.java:58)
at leviathan143.loottweaker.common.tweakers.loot.LootTableTweaker.onTableLoad(LootTableTweaker.java:51)
at net.minecraftforge.fml.common.eventhandler.ASMEventHandler_386_LootTableTweaker_onTableLoad_LootTableLoadEvent.invoke(.dynamic)
at net.minecraftforge.fml.common.eventhandler.ASMEventHandler.invoke(ASMEventHandler.java:90)
at net.minecraftforge.fml.common.eventhandler.EventBus.post(EventBus.java:179)
at net.minecraftforge.event.ForgeEventFactory.loadLootTable(ForgeEventFactory.java:653)
at net.minecraftforge.common.ForgeHooks.loadLootTable(ForgeHooks.java:1135)
at net.minecraft.world.storage.loot.LootTableManager$Loader.func_186518_c(LootTableManager.java:156)
at net.minecraft.world.storage.loot.LootTableManager$Loader.load(LootTableManager.java:72)
at net.minecraft.world.storage.loot.LootTableManager$Loader.load(LootTableManager.java:53)
at com.google.common.cache.LocalCache$LoadingValueReference.loadFuture(LocalCache.java:3716)
at com.google.common.cache.LocalCache$Segment.loadSync(LocalCache.java:2424)
at com.google.common.cache.LocalCache$Segment.lockedGetOrLoad(LocalCache.java:2298)
at com.google.common.cache.LocalCache$Segment.get(LocalCache.java:2211)
... 12 more
A detailed walkthrough of the error, its code path and all known details is as follows:
---------------------------------------------------------------------------------------
-- System Details --
Details:
Minecraft Version: 1.12
Operating System: Windows 8.1 (amd64) version 6.3
Java Version: 1.8.0_141, Oracle Corporation
Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation
Memory: 495334552 bytes (472 MB) / 1706180608 bytes (1627 MB) up to 4120510464 bytes (3929 MB)
JVM Flags: 8 total; -XX:SurvivorRatio=2 -XX:+DisableExplicitGC -XX:+UseConcMarkSweepGC -XX:+AggressiveOpts -XX:+UseStringDeduplication -XX:HeapDumpPath=MojangTricksIntelDriversForPerformance_javaw.exe_minecraft.exe.heapdump -Xms1024m -Xmx4096m
IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0
FML: MCP 9.40 Powered by Forge 14.21.1.2443 Optifine OptiFine_1.12_HD_U_C5 90 mods loaded, 90 mods active
States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored
UCHIJAA minecraft{1.12} [Minecraft] (minecraft.jar)
UCHIJAA mcp{9.19} [Minecraft Coder Pack] (minecraft.jar)
UCHIJAA FML{8.0.99.99} [Forge Mod Loader] (forge-1.12-14.21.1.2443-universal.jar)
UCHIJAA forge{14.21.1.2443} [Minecraft Forge] (forge-1.12-14.21.1.2443-universal.jar)
UCHIJAA mc64836coremod{0.1.1} [MC64836 Core Mod] (minecraft.jar)
UCHIJAA tnt_utilities_core{1.2.2} [TNTUtils Core] (minecraft.jar)
UCHIJAA additionalbanners{1.5.38} [Additional Banners] (AdditionalBanners-1.12-1.5.38.jar)
UCHIJAA forgeendertech{1.12-3.0.0.0} [Forge Endertech] (ForgeEndertech-1.12-3.0.0.0-build.0002.jar)
UCHIJAA adhooks{1.12-3.0.0.0} [Advanced Hook Launchers] (AdHooks-1.12-3.0.0.0-build.0002.jar)
UCHIJAA aiimprovements{0.0.1.3} [AI Improvements] (AIImprovements-1.12-0.0.1b3.jar)
UCHIJAA akashictome{1.2-9} [Akashic Tome] (AkashicTome-1.2-9.jar)
UCHIJAA antiqueatlas{4.4.4} [Antique Atlas] (antiqueatlas-1.12-4.4.4.jar)
UCHIJAA antiqueatlasoverlay{1.2} [Antique Atlas Overlay] (antiqueatlas-1.12-4.4.4.jar)
UCHIJAA astralsorcery{1.6.1} [Astral Sorcery] (astralsorcery-1.12-1.6.1.jar)
UCHIJAA quark{r1.2-104} [Quark] (Quark-r1.2-104.jar)
UCHIJAA autoreglib{1.3-13} [AutoRegLib] (AutoRegLib-1.3-13.jar)
UCHIJAA bedbugs{@VERSION@} [Bed Bugs] (BedBugs-1.12-1.0.1.jar)
UCHIJAA betterbuilderswands{0.11.1} [Better Builder's Wands] (BetterBuildersWands-1.12-0.11.1.245+69d0d70.jar)
UCHIJAA cyclopscore{0.10.12} [Cyclops Core] (CyclopsCore-1.12-0.10.12.jar)
UCHIJAA capabilityproxy{1.0.0} [CapabilityProxy] (CapabilityProxy-1.12-1.0.0.jar)
UCHIJAA carryon{1.1} [Carry On] (CarryOn_MC1.12_v1.1.jar)
UCHIJAA ceramics{1.12-1.3.2} [Ceramics] (Ceramics-1.12-1.3.2.jar)
UCHIJAA charcoal_pit{1.4_12} [Charcoal Pit] (CharcoalPit-[1.12]-1.4_12.jar)
UCHIJAA commoncapabilities{1.3.4} [CommonCapabilities] (CommonCapabilities-1.12-1.3.4.jar)
UCHIJAA crafttweaker{4.0.3} [CraftTweaker2] (CraftTweaker2-1.12-4.0.3.jar)
UCHIJAA ctgui{1.0.0} [CT-GUI] (CraftTweaker2-1.12-4.0.3.jar)
UCHIJAA jei{4.7.5.85} [Just Enough Items] (jei_1.12-4.7.5.85.jar)
UCHIJAA crafttweakerjei{2.0.0} [CraftTweaker JEI Support] (CraftTweaker2-1.12-4.0.3.jar)
UCHIJAA customizeddungeonloot{1.0.1} [§eCustomized Dungeon Loot] (Customized Dungeon Loot (v.1.0.1).jar)
UCHIJAA debugserverinfo{1.0.5} [DebugServerInfo] (DebugServerInfo-1.12-1.0.5.jar)
UCHIJAA degradeexplodedblocks{1.0.0} [DegradeExplodedBlocks] (degradeexplodedblocks-1.11.2-1.0.0.jar)
UCHIJAA dirt2path{1.7.0} [Dirt2Path] (dirt2path-1.7.0.jar)
UCHIJAA doggytalents{1.14.2.295} [Doggy Talents] (DoggyTalents-1.12-1.14.2.295-universal.jar)
UCHIJAA dsurround{3.4.6.3} [§3Dynamic Surroundings] (DynamicSurroundings-1.12-3.4.6.3.jar)
UCHIJAA presets{3.4.6.3} [§3Presets!] (DynamicSurroundings-1.12-3.4.6.3.jar)
UCHIJAA enchantingbottles{1.0} [Enchanting Bottles] (enchantingbottles-1.0.jar)
UCHIJAA csb_ench_table{1.1.2-beta2} [Lapis Stays in the Enchanting Table] (EnchantingTable-1.12-1.1.2-beta2.jar)
UCHIJAA enchdesc{1.0.4} [Enchantment Descriptions] (EnchantmentDescriptions-1.12-1.0.4.jar)
UCHIJAA equal_dragons{1.0} [EqualDragons] (EqualDragons-1.0.jar)
UCHIJAA tschipplib{1.1} [TschippLib] (TschippLib MC1.12 v1.1.jar)
UCHIJAA extraambiance{1.0.1} [Extra Ambiance] (ExtraAmbiance MC1.12 v1.0.1.jar)
UCHIJAA fairylights{2.1.1} [Fairy Lights] (fairylights-2.1.1-1.12.jar)
UCHIJAA friendlyfire{1.5.5} [Friendly Fire] (FriendlyFire-1.12-1.5.5.jar)
UCHIJAA mcmultipart{2.2.2} [MCMultiPart] (MCMultiPart-2.2.2.jar)
UCHIJAA ftbl{0.0.0} [FTBLib] (FTBLib-4.0.5.jar)
UCHIJAA ftbu{0.0.0} [FTBUtilities] (FTBUtilities-4.0.3.jar)
UCHIJAA gravestone{1.8.4} [Gravestone Mod] (gravestone-1.8.4.jar)
UCHIJAA hopperducts{1.5} [Hopper Ducts] (hopperducts-mc1.12-1.5.jar)
UCHIJAA incontrol{3.6.0} [InControl] (incontrol-1.12-3.6.0.jar)
UCHIJAA integrateddynamics{0.8.4} [Integrated Dynamics] (IntegratedDynamics-1.12-0.8.4.jar)
UCHIJAA integrateddynamicscompat{1.0.0} [IntegratedDynamics-Compat] (IntegratedDynamics-1.12-0.8.4.jar)
UCHIJAA jepb{1.2} [Just Enough Pattern Banners] (jepb-1.12-1.2.jar)
UCHIJAA jaff{1.7_for_1.12} [Just a Few Fish] (JustAFewFish-1.7_for_1.12.jar)
UCHIJAA loottweaker{0.0.6.3} [LootTweaker] (LootTweaker-1.12-0.0.6.3.jar)
UCHIJAA jeresources{0.8.3.23} [Just Enough Resources] (JustEnoughResources-1.12-0.8.3.23.jar)
UCHIJAA kleeslabs{5.3.2} [KleeSlabs] (KleeSlabs_1.12-5.3.2.jar)
UCHIJAA lostcities{0.0.20beta2} [The Lost Cities] (lostcities-1.12-0.0.20beta2.jar)
UCHIJAA lostsouls{0.0.2beta} [Lost Souls] (lostsouls-1.12-0.0.2beta.jar)
UCHIJAA malisiscore{1.12-6.0.2} [MalisisCore] (malisiscore-1.12-6.0.2.jar)
UCHIJAA malisisblocks{1.12-6.0.0} [Malisis Blocks] (malisisblocks-1.12-6.0.0.jar)
UCHIJAA manawell{0.0.2} [Mana Wells] (manawell-1.12--0.0.2.jar)
UCHIJAA mendingfix{1.0.1} [Mending Fix] (mendingfix-1.0.1.jar)
UCHIJAA netherchest{0.2.1} [Nether Chest] (netherchest-0.2.1.jar)
UCHIJAA nex{2.1.11.12} [NetherEx] (NetherEx-1.12-2.1.11.12.jar)
UCHIJAA nonausea{1.0} [NoNausea] (NoNausea-MC1.12-1.0.jar)
UCHIJAA samsocean{1.0.1} [Ocean Floor Classic] (OceanFloor-1.12-1.0.1.jar)
UCHIJAA passableleaves{6.1.0.0} [Passable Leaves] (PassableLeaves-1.12-6.1.0.0.jar)
UCHIJAA progressivedifficulty{1.12_1.3.1} [Progressive Difficulty] (ProgressiveDifficulty-1.12_1.3.1.jar)
UCHIJAA quickleafdecay{1.2.3} [Quick Leaf Decay] (QuickLeafDecay-MC1.12-1.2.3.jar)
UCHIJAA reauth{3.5.1} [ReAuth] (ReAuth-3.5.1.jar)
UCHIJAA ruins{16.7} [Ruins Mod] (Ruins-1.12.jar)
UCHIJAA rustic{0.4.1} [Rustic] (rustic-0.4.1.jar)
UCHIJAA saltmod{1.12_d} [Salty Mod] (SaltyMod_1.12_d.jar)
UCHIJAA scannable{1.5.0.9} [Scannable] (Scannable-MC1.12-1.5.0.9.jar)
UCHIJAA skyblocks{0.1.0-beta-1.12} [Skyblocks] (Skyblocks-0.1.0-beta-1.12.jar)
UCHIJAA soundfilters{0.10_for_1.12} [Sound Filters] (SoundFilters-0.10_for_1.12.jar)
UCHIJAA stepup{4.0-mc1.12} [StepUp] (StepUp-4.0-mc1.12.jar)
UCHIJAA stg{1.12-1.2.1} [SwingThroughGrass] (stg-1.12-1.2.1.jar)
UCHIJAA structuredcrafting{0.1.8} [Structured Crafting] (StructuredCrafting-1.12-0.1.8.jar)
UCHIJAA stupidthings{1.1.3} [Stupid Things] (Stupid Things-1.12-1.1.3.jar)
UCHIJAA tis3d{1.2.2.5} [TIS-3D] (TIS-3D-MC1.12-1.2.2.5.jar)
UCHIJAA tnt_utilities{1.2.2} [TNTUtils] (tnt_utilities-mc1.12-1.2.2.jar)
UCHIJAA toolbelt{1.7.1} [Tool Belt] (ToolBelt-1.12.0-1.7.1.jar)
UCHIJAA villagenames{1.11.2-1.1a} [Village Names] (VillageNames-1.12-1.1b.jar)
UCHIJAA vtweaks{1.4.11.3} [V-Tweaks] (VTweaks-1.12-1.4.11.3.jar)
UCHIJAA wearablebackpacks{3.0.4} [Wearable Backpacks] (WearableBackpacks-1.12-3.0.4.jar)
UCHIJAA winterwonderland{1.2.0} [winterwonderland] (WinterWonderLand-1.2.0.jar)
UCHIJAA withsprinkles{1.08} [With Sprinkles] (With Sprinkles-1.08.jar)
UCHIJAA gravelores{1.3} [Gravel Ores] (gravelores-1.7b.jar)
UCHIJAA mbm{1.9.0.3} [Master Blocks Mod] (mbm-Master_Blocks_Mod_1.9.0.3-04S_1.12.jar)
Loaded coremods (and transformers):
PLCore (PassableLeaves-1.12-6.1.0.0.jar)
passableleaves.core.PLTransformer
LoadingPlugin (Quark-r1.2-104.jar)
vazkii.quark.base.asm.ClassTransformer
AstralCore (astralsorcery-1.12-1.6.1.jar)
TNTUtilities Core (tnt_utilities-mc1.12-1.2.2.jar)
ljfa.tntutils.asm.ExplosionTransformer
MalisisCorePlugin (malisiscore-1.12-6.0.2.jar)
net.malisis.core.util.chunkcollision.ChunkCollisionTransformer
net.malisis.core.util.chunkblock.ChunkBlockTransformer
net.malisis.core.renderer.transformer.MalisisRendererTransformer
net.malisis.core.renderer.icon.asm.TextureMapTransformer
net.malisis.core.util.clientnotif.ClientNotifTransformer
MC64836CoreMod (mc64836-0.1.1.jar)
nge.sse.mc64836.EntityMinecartTransformer
DummyPlugin (ForgeEndertech-1.12-3.0.0.0-build.0002.jar)
LoadingPlugin (NoNausea-MC1.12-1.0.jar)
lumien.nonausea.asm.ClassTransformer
DynamicSurroundingsCore (DynamicSurroundings-1.12-3.4.6.3.jar)
org.blockartistry.DynSurround.asm.Transformer
Profiler Position: N/A (disabled)
Player Count: 0 / 8; []
Type: Integrated Server (map_client.txt)
Is Modded: Definitely; Client brand changed to 'fml,forge'
I'm not sure what's happening here. The loot tables/pools should not be frozen at this point. The only thing I can think of is that another mod could be firing LootTableLoadEvent after tables/pools are frozen, or freezing tables/pools early. I'm working on some tweaks to LootTweaker that should make any culprit easier to find.
I also have In Control! and Customized Dungeon Loot in the pack, which can both manipulate loot tables. I don't have any loot manipulations in the In Control! configs, but I've left the defaults in Customized Dungeon Loot.
Edit to add that I just tried loading into a world without these two mods and it still happened. I know there are a few other mods in the pack that add things to loot tables though.
Oh, also your script has incorrect syntax.
There's no overload of addItemEntry
with the parameters IItemStack, int, int, LootFunction[], LootCondition[]
. You need to use addItemEntryHelper
since you're using the helper classes. If you were using JSON strings, you'd need to use addItemEntryJSON
Thanks for pointing that out, I had intended to remove the conditions. Having done so, I'm still getting the same error though.
I've just managed to reproduce this. It doesn't happen for the first world loaded after MC startup, but any worlds loaded after will have the issue.
@Tetrajak
Try this test build, it should fix the issue. If it works I'll make a proper release on CurseForge with the fix.