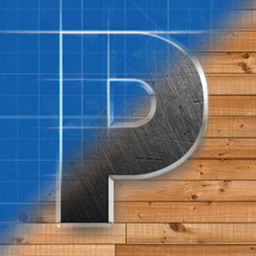
Structures are bypassing protections
LemADEC opened this issue ยท 8 comments
As of prefab-1.1.1.1, users with no permissions can grief protected areas from plugins, or spawn itself using the prefab structures.
Here's a couple snipet you can use to do those checks:
IBlockState blockState = world.getBlockState(blockPosEvent);
if (!blockState.getBlock().isAir(blockState, world, blockPosEvent)) {
BlockEvent.BreakEvent breakEvent = new BlockEvent.BreakEvent(
world, blockPosEvent, world.getBlockState(blockPosEvent),
entityPlayer);
MinecraftForge.EVENT_BUS.post(breakEvent);
return breakEvent.isCanceled();
}
and
BlockEvent.PlaceEvent placeEvent = new BlockEvent.PlaceEvent(
new BlockSnapshot(world, blockPosEvent, blockState), Blocks.AIR.getDefaultState(),
entityPlayer );
MinecraftForge.EVENT_BUS.post(placeEvent);
return placeEvent.isCanceled();
As of 1.7.10, when replacing a block, you need to do both the break and place check.
I think in 1.10 you can check a whole area in a single call to save resources.
Thanks for bringing this to my attention. Really wasn't thinking about this unfortunately.
I do have a way to get the entire area which will be cleared and set.
I will do some research on how to make this efficient.
It might take me a day or two to get this completed and tested properly.
Sounds good. I assume those check and placement are split over several ticks so it doesn't lag out the server?
The building is done in a few different phases.
The first part of building is to clear out the entire area in order to be prepared for the replacement blocks. This also allows a player to dig inside a mountain but still end up with a usable building instead of just a bunch of different blocks withing the stone :). All of that is done in 1 tick and doesn't slow things down too much since everything is being replaced with Air and as such there isn't a lot of physics/redstone states to process. I am planning on adding these new checks before this clearing occurs so it will be up to the mod authors to help make this process efficient.
The next part is where the structure specific blocks are placed, these are placed 100 blocks per tick. This part is staggered because this part does lag down the server. 100 blocks are placed per structure per tick per player. So Player "A" has their 100 blocks placed and then Player "B" has their 100 blocks placed for the tick. This continues until there are no longer any blocks to place for the structure and player.
What I am planning on implementing is the break/place checks before the structure space is cleared. I will be checking all block positions, if any of the blocks return a false for that event then the entire structure cannot be placed. I don't want partial structures generated.
Since it can take a few seconds for a structure to be generated once it's initiated, a protected block could be replaced if the timing is just right. I think this is the best approach to prevent the replacement of protected spaces.
Removing block is placing air block which is effectively the same as placing block, you don't want to clear the whole area in one tick. I would suggest to limit to 100 per tick per player.